1.0.0
General
RuStore In-app updates SDK enable users to be kept up to date with the latest app version on their device. This allows them to stay informed about any performance enhancements or bug fixes that have been implemented.
User scenario example
Additionally, the SDK offers the ability to notify users of a new version and provide an option to install it. The installation process can occur in the background, while the user can track the progress of the update.
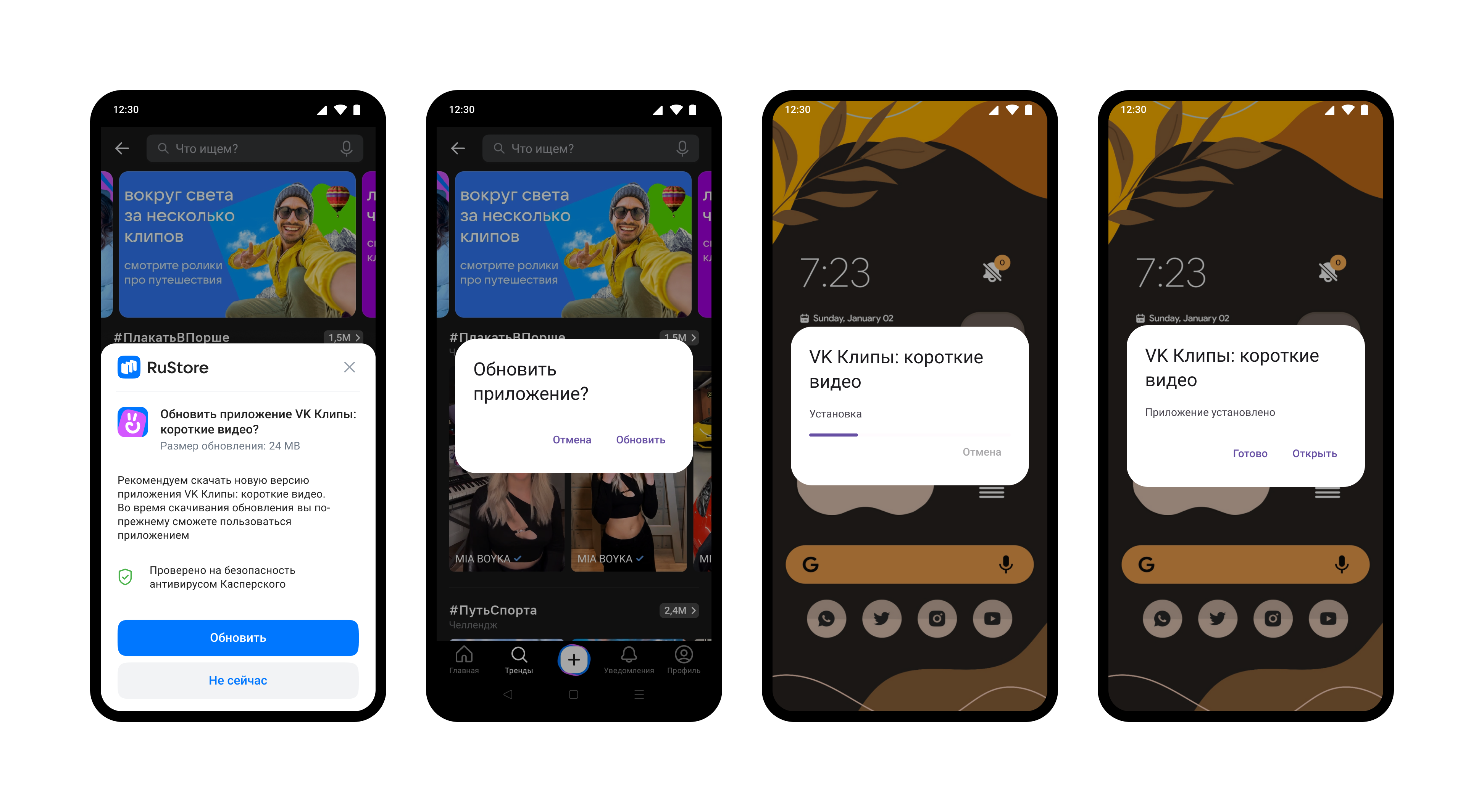
Prerequisites
For RuStore In-app updates SDK to operate correctly, the following conditions need to be met:
- Android 7.0 or later.
- RuStore app version on the device is up-to-date.
- User is authorized in RuStore.
- RuStore app is allowed to install applications.
Connecting to project
To get started, follow these steps:
- Copy the contents of the
Plugins
folder from the official RuStore repository on gitflic toPlugins
folder of your project. - Restart Unreal Engine.
- In the plug-in list (Edit > Plugins > Project > Mobile) select RuStoreAppUpdate and RuStoreCore.
- Enable
RuStoreCore
andRuStoreAppUpdate
inYourProject.Build.cs
in the listPublicDependencyModuleNames
. - In the project settings (Edit → Project Settings → Android) set the
Minimum SDK Version
parameter at least to24
andTarget SDK Version
parameter to31
or later.
Create update manager
Create update manager before calling library methods.
How to initialize a library
Initialize the library before calling its methods.
URuStoreAppUpdateManager::Instance()->Init();
All operations with the client are also accessible from Blueprints. Below is an initialization example.
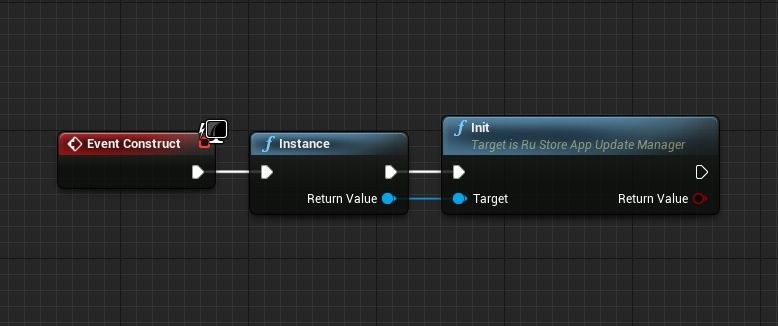
The Init
call ties the object to the scene root, and, If no further work with the object is needed, execute the Dispose
method to free memory. The Dispose
method call will untie the object from root and securely complete all sent requests.
Deinitialization
URuStoreAppUpdateManager::Instance()->Dispose();
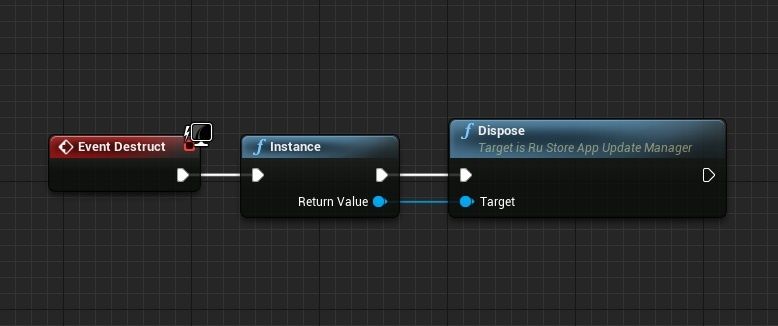
Initialization check
If you need to check whether the library is initialized, use the GetIsInitialized
method. Method returns a bool
value type:
true
— if the library is initialised;false
— ifInit
has not yet been called.
bool bIsInitialized = URuStoreAppUpdateManager::Instance()->GetIsInitialized();
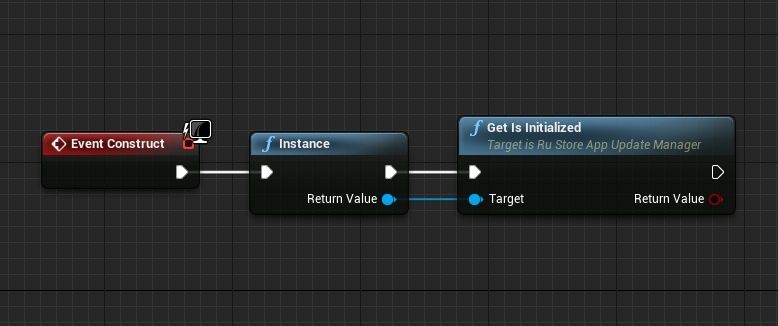
Check for updates
Before requesting an update, check if it is available for your application To check for updates, call the GetAppUpdateInfo
method. When this method is called, the following conditions will be verified:
- The current version of RuStore is installed on the user's device.
- The user and the app are not banned in RuStore.
- RuStore app is allowed to install applications.
- User is authorized in RuStore.
Upon calling this method, the FURuStoreAppUpdateInfo
object will be returned which contains information regarding any required updates. It is recommended to request and cache this object in advance, ensuring a prompt and convenient update download process for the user.
GetAppUpdateInfo
request returns requestId
, that is unique within a single application run. Each event returns requestId
of the request that triggered the event.
long requestId = GetAppUpdateInfo(
[](long requestId, TSharedPtr<FURuStoreAppUpdateInfo, ESPMode::ThreadSafe> response) {
// Process response
},
[](long requestId, TSharedPtr<FURuStoreError, ESPMode::ThreadSafe> error) {
// Process error
}
);
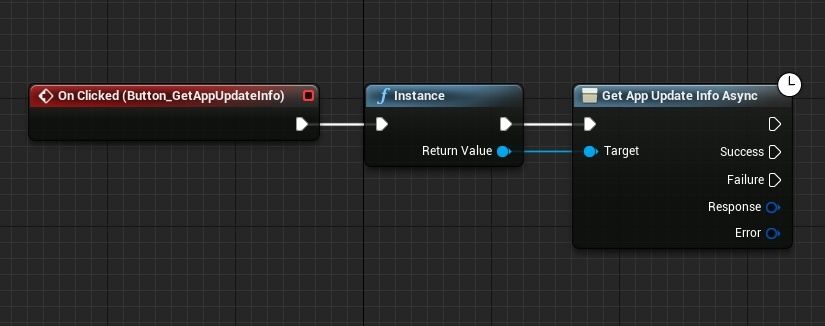
The Success
callback notification returns a FURuStoreAppUpdateInfo
structure in the Response
parameter. The structure contains a set of parameters required to determine the update availability.
USTRUCT(BlueprintType)
struct RUSTOREAPPUPDATE_API FURuStoreAppUpdateInfo
{
GENERATED_USTRUCT_BODY()
FURuStoreAppUpdateInfo()
{
updateAvailability = EURuStoreUpdateAvailability::UNKNOWN;
installStatus = EURuStoreInstallStatus::UNKNOWN;
availableVersionCode = 0;
}
UPROPERTY(BlueprintReadOnly)
EURuStoreUpdateAvailability updateAvailability;
UPROPERTY(BlueprintReadOnly)
EURuStoreInstallStatus installStatus;
UPROPERTY(BlueprintReadOnly)
int64 availableVersionCode;
};
-
:updateAvailability
— update availability:UNKNOWN (uint8 = 0)
— by default.;UPDATE_NOT_AVAILABLE (uint8 = 1)
— no update required.;UPDATE_AVAILABLE (uint8 = 2)
— update needs to be downloaded or it has already been downloaded to the user's device.;DEVELOPER_TRIGGERED_UPDATE_IN_PROGRESS (uint8 = 3)
— update is already being downloaded or installation is already running..
-
:installStatus
— update installation status, if the user has already started the update installation at the time:UNKNOWN (uint8 = 0)
— by default.;DOWNLOADED (uint8 = 1)
— successfully downloaded.;DOWNLOADING (uint8 = 2)
— currently being downloaded.;FAILED (uint8 = 3)
— error.;PENDING (uint8 = 5)
— awaiting update..
-
availableVersionCode
— update version code.
The update download is only available if the updateAvailability
field has the UPDATE_AVAILABLE
value.
Failure
callback returns структуру with the error information. Error structure is described in Error Handling.
Download and install updates
Using listener
After the update's availability is confirmed, you can receive the update's download status in OnStateUpdatedInstanceEvent
of the URuStoreAppUpdateManager
object.
DECLARE_DYNAMIC_MULTICAST_DELEGATE_TwoParams(FRuStoreOnStateUpdatedInstanceDelegate, int64, listenerId, FURuStoreInstallState, state);
UPROPERTY(BlueprintAssignable, Category = "RuStore AppUpdate Manager")
FRuStoreOnStateUpdatedInstanceDelegate OnStateUpdatedInstanceEvent;
FScriptDelegate Delegate;
Delegate.BindUFunction(YourUObjectPtr, FName("YourCallbackMethod"));
URuStoreAppUpdateManager::Instance()->OnStateUpdatedInstanceEvent.Add(Delegate);
UFUNCTION()
void YourCallbackMethod(int64 listenerId, FURuStoreInstallState state) {
// Process callback
}
Subscribe to a listener event from Blueprint:
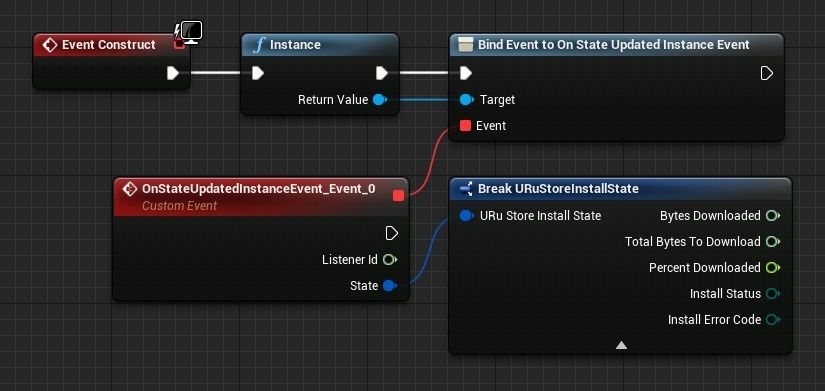
The OnStateUpdatedInstanceEvent
event returns a FURuStoreInstallState
object in the state
parameter describing the current download status. FURuStoreInstallState
is described in Checking the update download status.
Listener interface
The URuStoreAppUpdateManager
class implements a standard listener. You can create your own listener class using the IRuStoreInstallStateUpdateListenerInterface
interface.
UINTERFACE(Blueprintable)
class RUSTOREAPPUPDATE_API URuStoreInstallStateUpdateListenerInterface : public UInterface
{
GENERATED_BODY()
};
class IRuStoreInstallStateUpdateListenerInterface
{
GENERATED_BODY()
public:
UFUNCTION(BlueprintCallable, BlueprintNativeEvent, Category = "RuStore InstallStateUpdate Listener Interface")
void OnStateUpdated(int64 listenerId, FURuStoreInstallState& state);
};
The OnStateUpdated
event returns a FURuStoreInstallState
object in the state
parameter describing the current download status. FURuStoreInstallState
is described in Checking the update download status.
Calling the RegisterListener
method performs registering the listener.
For the default URuStoreAppUpdateManager
listener, calling RegisterListener
is not required.
int64 listenerId = URuStoreAppUpdateManager::Instance()->RegisterListener(YourListenerPtr);
YourListenerPtr
— object of a class that implements IRuStoreInstallStateUpdateListenerInterface
.
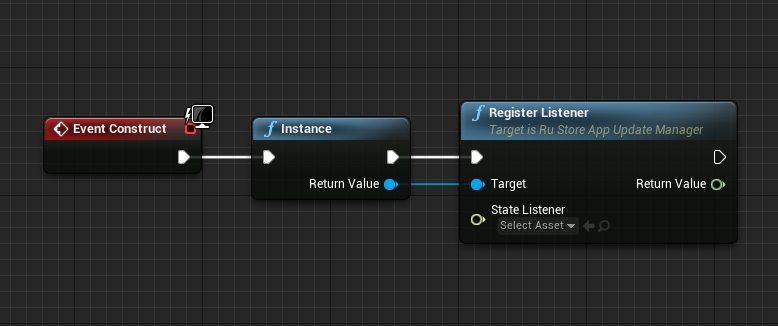
Check update status
FURuStoreInstallState
describes the current download status.
USTRUCT(BlueprintType)
struct RUSTOREAPPUPDATE_API FURuStoreInstallState
{
GENERATED_USTRUCT_BODY()
FURuStoreInstallState()
{
bytesDownloaded = 0;
totalBytesToDownload = 0;
percentDownloaded = 0;
installStatus = EURuStoreInstallStatus::UNKNOWN;
installErrorCode = EURuStoreInstallErrorCode::ERROR_UNKNOWN;
}
UPROPERTY(BlueprintReadWrite)
int64 bytesDownloaded;
UPROPERTY(BlueprintReadWrite)
int64 totalBytesToDownload;
UPROPERTY(BlueprintReadWrite)
float percentDownloaded;
UPROPERTY(BlueprintReadWrite)
EURuStoreInstallStatus installStatus;
UPROPERTY(BlueprintReadWrite)
EURuStoreInstallErrorCode installErrorCode;
};
bytesDownloaded
— number of bytes downloaded.totalBytesToDownload
— total number of bytes that need to be downloaded.;percentDownloaded
— update download progress in percent;installStatus
— update installation status, if the user has already started the update installation at the time:UNKNOWN (int == 0)
— default status;DOWNLOADED (int == 1)
— downloaded;DOWNLOADING (int == 2)
— being downloaded;FAILED (int == 3)
— error;PENDING (Int == 5)
— waiting for download.
installErrorCode
— error code during download. All possible errors are described in Error Handling.
Delete listener
If you no longer need a listener, use the UnregisterListener
method to remove a listener, passing a previously registered listener to the method. UnregisterListener
must be called for all listeners before the application terminates.
For the default URuStoreAppUpdateManager
listener, it is not necessary to call UnregisterListener
on application shutdown.
bool bIsDone = URuStoreAppUpdateManager::Instance()->UnregisterListener(YourListenerPtr);
YourListenerPtr
— object of a class that implements IRuStoreInstallStateUpdateListenerInterface
.
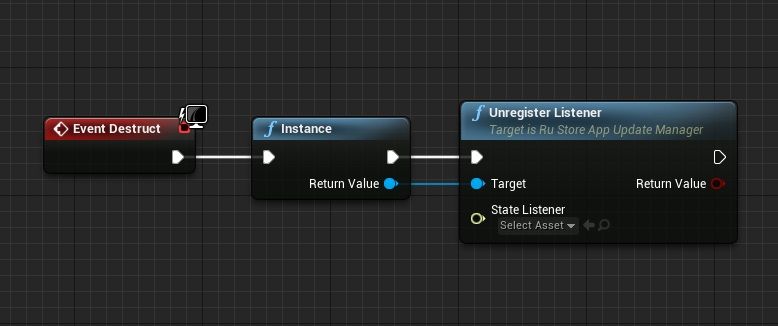
If you implement your own listener, you can also unregister the default listener to save resources.
auto instance = URuStoreAppUpdateManager::Instance();
bool bIsDone = instance->UnregisterListener(instance);
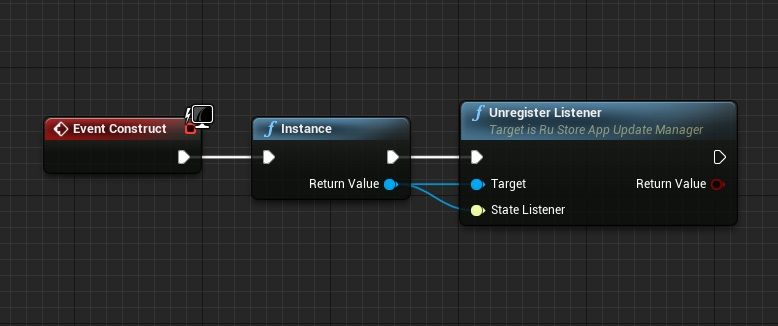
Start downloading update
Delayed update
Running an upgrade script
Update with RuStore UI
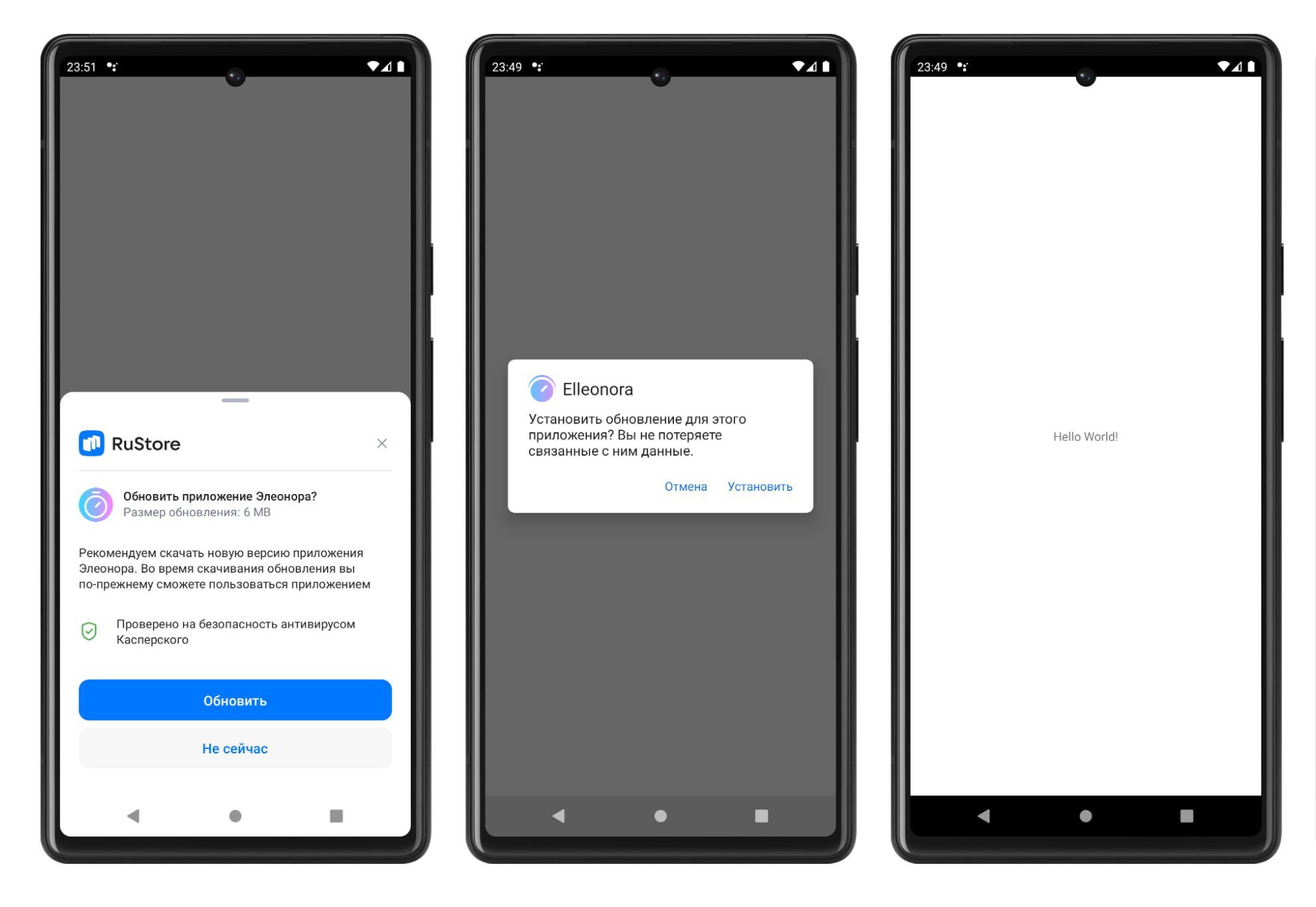
- To confirm the update, the user is presented with a RuStore UI dialogue.
- When you click the Update button, a dialogue box will appear to confirm that the update has been installed.
- If the update is successfully installed, the application will be closed.
Running an upgrade script
Use the StartUpdateFlow
method to start downloading the application update.
The FURuStoreAppUpdateInfo
object becomes invalid after a single use. To call the StartUpdateFlow
method again, request FURuStoreAppUpdateInfo
again using the GetAppUpdateInfo
method.
See Checking for updates.
StartUpdateFlow
request returns requestId
, that is unique within a single application run. Each event returns requestId
of the request that triggered the event.
EURuStoreAppUpdateOptions appUpdateOptions = EURuStoreAppUpdateOptions::DELAYED;
long requestId = StartUpdateFlow(
appUpdateOptions,
[](long requestId, EURuStoreUpdateFlowResult response) {
// Process response
},
[](long requestId, TSharedPtr<FURuStoreError, ESPMode::ThreadSafe> error) {
// Process error
}
);
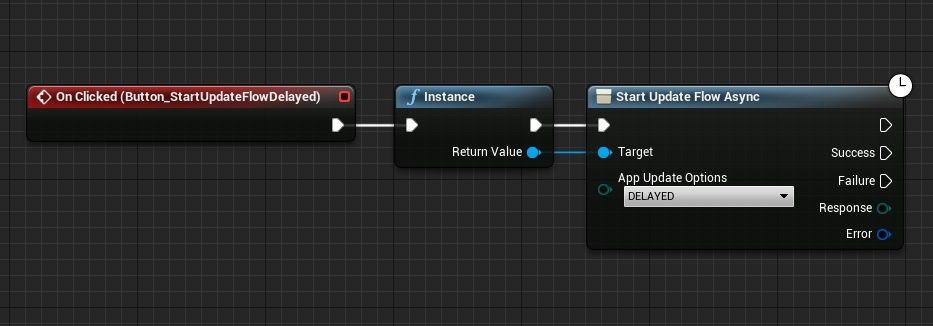
appUpdateOptions
— update type:DELAYED
— delayed update. To confirm the update, the user is presented with a RuStore UI dialogue.SILENT
— silent update. The update will be downloaded in the background.IMMEDIATE
— forced update. Until the update is installed, use of the application will be blocked.
TheSuccess
callback returns EURuStoreUpdateFlowResult
in the Response
parameter:
EURuStoreUpdateFlowResult::RESULT_OK
— user has confirmed the download.EURuStoreUpdateFlowResult::RESULT_CANCELED
— user refused to install the update.
The Failure
callback returns the FURuStoreError
structure with the error information in the Error
parameter. The structure of the FURuStoreError
error is described in Error Handling.
After calling the StartUpdateFlow
method you can monitor the download status in the OnStateUpdatedInstanceEvent
event.
Once the EURuStoreInstallStatus::DOWNLOADED
status is received in the installStatus
field of FURuStoreInstallState
, the update installation method can be called.
Forced update
Running an upgrade script
Update with RuStore UI
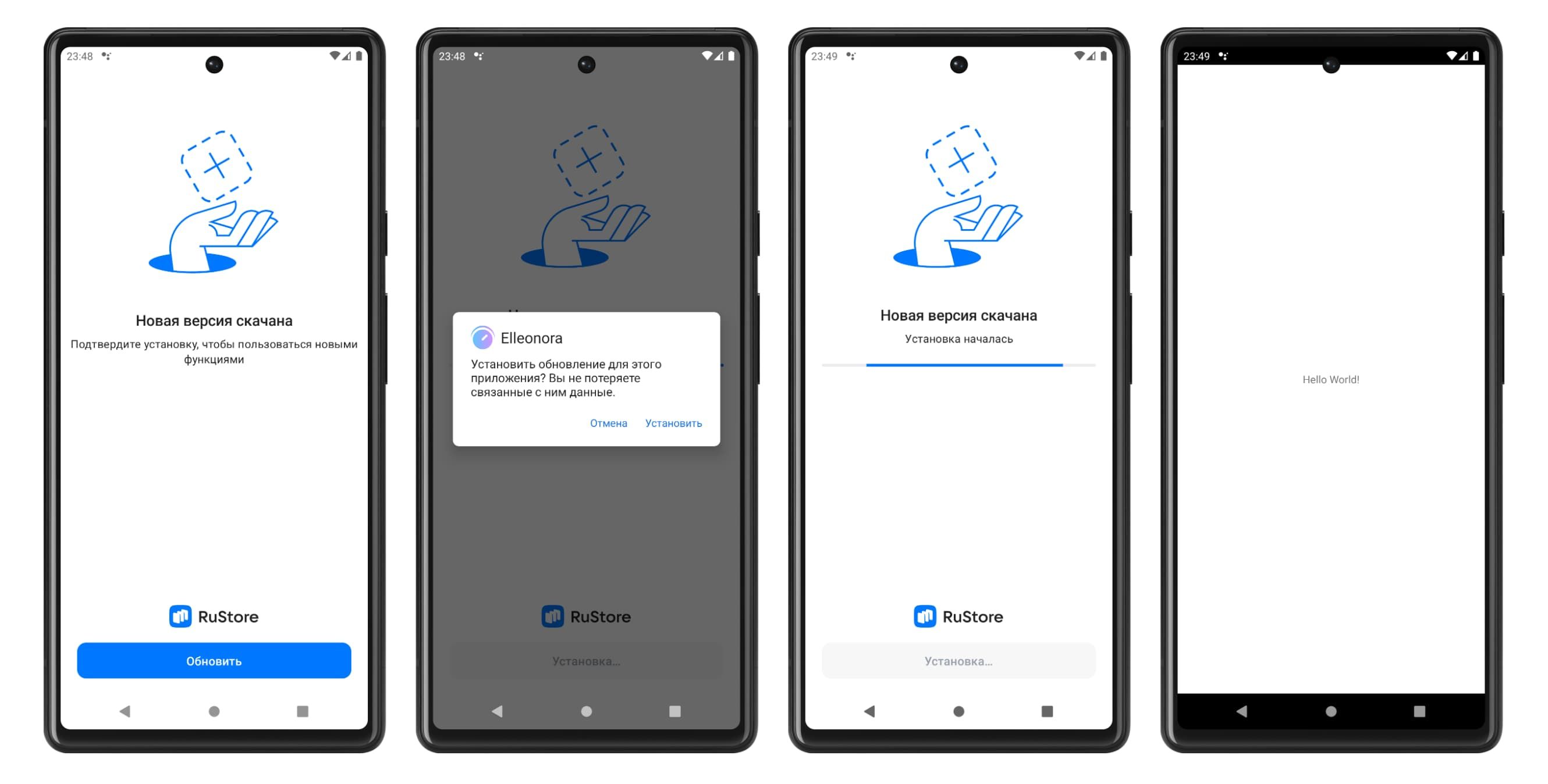
- To confirm the update, the user is presented with a RuStore UI dialogue. Until the update is installed, use of the application will be blocked.
- When you click the Update button, a dialogue box will appear to confirm that the update has been installed.
- If you then click on the 'Install' button, a full-screen dialogue box will appear asking you to install the new app version.
- Once the installation is complete, the application will restart.
If the Rustore version is 1.37 or higher, the application will restart. If the version of Rustore is lower, the application will close to install the update. It will not reopen when the update is complete.
Running an upgrade script
Once you receive FURuStoreAppUpdateInfo
, you can check if the forced update is available.
bool bIsAvailable = URuStoreAppUpdateManager::Instance()->CheckIsImmediateUpdateAllowed();
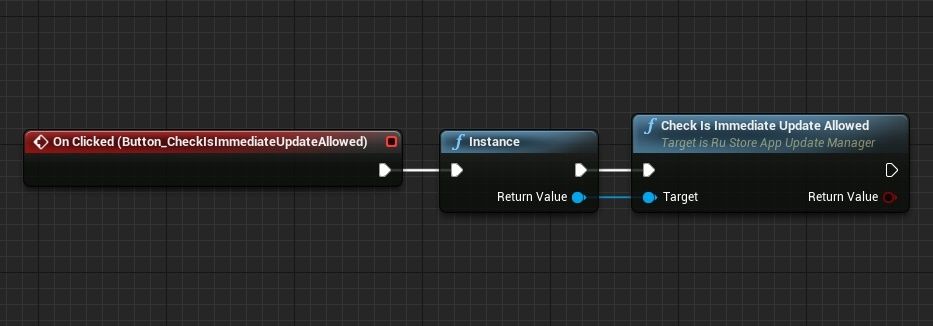
The result of CheckIsImmediateUpdateAllowed
is recommended when deciding whether to run a forced update, but this result does not affect the ability to run the script. Your internal app logic determines the necessity to run the update script.
Use the StartUpdateFlow
method to start downloading the application update.
The FURuStoreAppUpdateInfo
object becomes invalid after a single use. To call the StartUpdateFlow
method again, request FURuStoreAppUpdateInfo
again using the GetAppUpdateInfo
method.
See Checking for updates.
StartUpdateFlow
request returns requestId
, that is unique within a single application run. Each event returns requestId
of the request that triggered the event.
EURuStoreAppUpdateOptions appUpdateOptions = EURuStoreAppUpdateOptions::IMMEDIATE;
long requestId = StartUpdateFlow(
appUpdateOptions,
[](long requestId, EURuStoreUpdateFlowResult response) {
// Process response
},
[](long requestId, TSharedPtr<FURuStoreError, ESPMode::ThreadSafe> error) {
// Process error
}
);
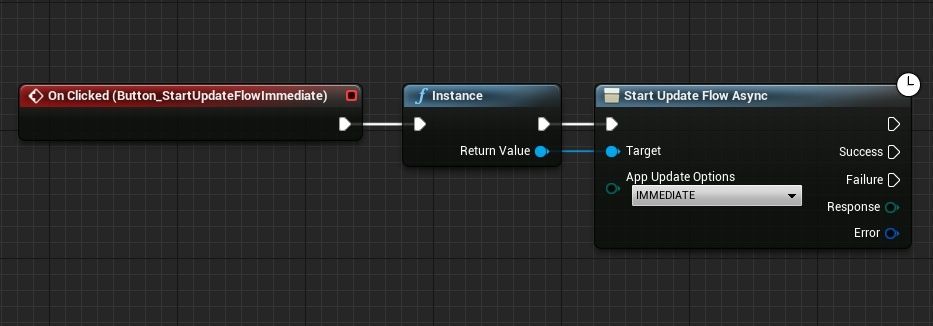
appUpdateOptions
— update type:DELAYED
— delayed update. To confirm the update, the user is presented with a RuStore UI dialogue.SILENT
— silent update. The update will be downloaded in the background.IMMEDIATE
— forced update. Until the update is installed, use of the application will be blocked.
TheSuccess
callback returns EURuStoreUpdateFlowResult
in the Response
parameter:
EURuStoreUpdateFlowResult::RESULT_OK (-1)
— update completed, the code may not be received as the app terminates at the time of update.EURuStoreUpdateFlowResult::RESULT_CANCELED
— flow interrupted by the user or an error occurred. When you receive this code, you are expected to exit the app.EURuStoreUpdateFlowResult::ACTIVITY_NOT_FOUND
— RuStore not installed, or the installed version does not support immediate updates (RuStore versionCode
<191
).
The Failure
callback returns the FURuStoreError
structure with the error information in the Error
parameter. The structure of the FURuStoreError
error is described in Error Handling.
No further action is required if the update is successful.
Silent update
Silent Update Scenario Description
Update without RuStore UI
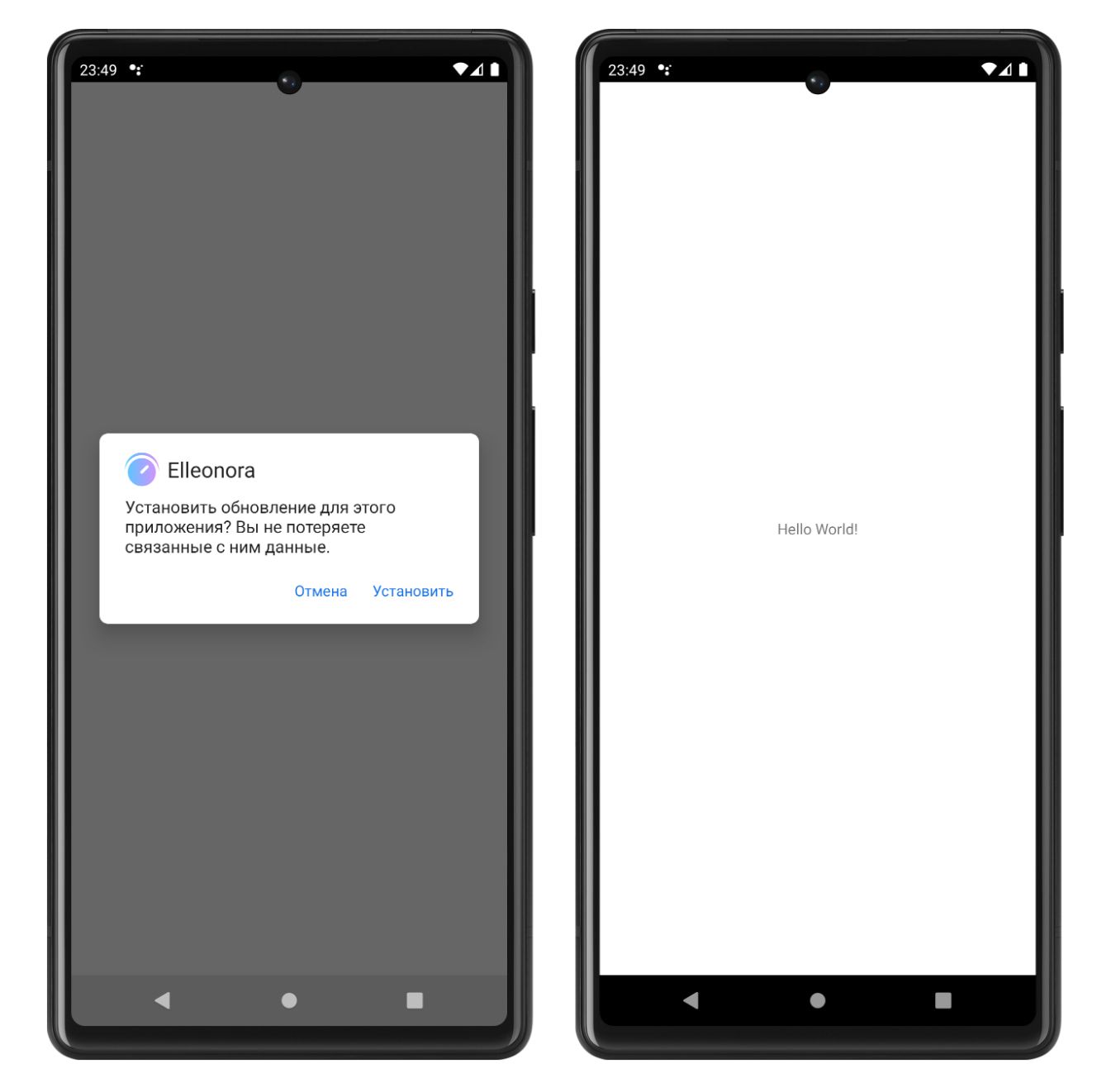
- The user will be presented with a dialogue box to confirm the installation of the update (the update will be downloaded in the background).
- If the update is successfully installed, the application will be closed.
Running an upgrade script
Use the StartUpdateFlow
method to start downloading the application update.
The FURuStoreAppUpdateInfo
object becomes invalid after a single use. To call the StartUpdateFlow
method again, request FURuStoreAppUpdateInfo
again using the GetAppUpdateInfo
method.
See Checking for updates.
StartUpdateFlow
request returns requestId
, that is unique within a single application run. Each event returns requestId
of the request that triggered the event.
EURuStoreAppUpdateOptions appUpdateOptions = EURuStoreAppUpdateOptions::SILENT;
long requestId = StartUpdateFlow(
appUpdateOptions,
[](long requestId, EURuStoreUpdateFlowResult response) {
// Process response
},
[](long requestId, TSharedPtr<FURuStoreError, ESPMode::ThreadSafe> error) {
// Process error
}
);
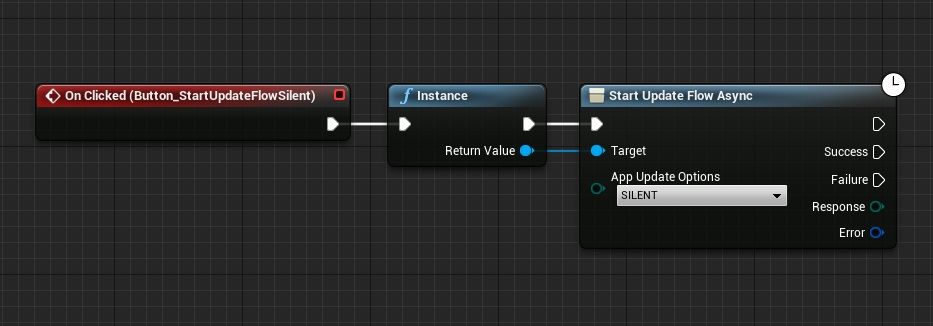
appUpdateOptions
— update type:DELAYED
— delayed update. To confirm the update, the user is presented with a RuStore UI dialogue.SILENT
— silent update. The update will be downloaded in the background.IMMEDIATE
— forced update. Until the update is installed, use of the application will be blocked.
TheSuccess
callback returns EURuStoreUpdateFlowResult
in the Response
parameter:
EURuStoreUpdateFlowResult::RESULT_OK
— task to download the update has been registered.
The Failure
callback returns the FURuStoreError
structure with the error information in the Error
parameter. The structure of the FURuStoreError
error is described in Error Handling.
After calling the StartUpdateFlow
method you can monitor the download status in the OnStateUpdatedInstanceEvent
event.
Once the EURuStoreInstallStatus::DOWNLOADED
status is received in the installStatus
field of FURuStoreInstallState
, the update installation method can be called.
Silent update requires a separate interface to operate.
Update installation
It is advisable to notify the user that the update is prepared for installation at this point.
Use the CompleteUpdate
method to start the update installation. The update is carried out through the native android tool. If the update is successfully installed, the application will be closed.
requestId = CompleteUpdate(
[](long requestId, TSharedPtr<FURuStoreError, ESPMode::ThreadSafe> error) {
// Process error
}
);
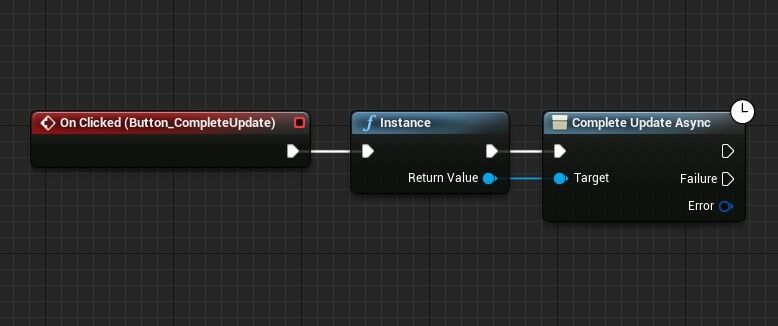
The Failure
callback returns the FURuStoreError
structure with the error information in the Error
parameter. The structure of the FURuStoreError
error is described in Error Handling.
Errors processing
It is not recommended to display the error to the user yourself if you get onFailure
in response. It can negatively affect the user experience.
USTRUCT(BlueprintType)
struct RUSTORECORE_API FURuStoreError
{
GENERATED_USTRUCT_BODY()
FURuStoreError()
{
name = "";
description = "";
}
UPROPERTY(BlueprintReadOnly)
FString name;
UPROPERTY(BlueprintReadOnly)
FString description;
};
name
—simpleName
.description
— error description.
Possible errors
RuStoreNotInstalledException
— RuStore is not installed on the user's device;RuStoreOutdatedException
— RuStore version installed on the user's device does not support this SDK;RuStoreUserUnauthorizedException
— user is not authorized in RuStore;RuStoreException
— basic RuStore error from which other errors are inherited;RuStoreInstallException(public val code: Int)
— download and installation error.ERROR_UNKNOWN(Int = 4001)
— unknown error.ERROR_DOWNLOAD(Int = 4002)
— error while downloading.ERROR_BLOCKED(Int = 4003)
— installation blocked by system.ERROR_INVALID_APK(Int = 4004)
— invalid update APK.ERROR_CONFLICT(Int = 4005)
— conflict with the current app version.ERROR_STORAGE(Int = 4006)
— insufficient device storage.ERROR_INCOMPATIBLE(Int = 4007)
— incompatible with device.ERROR_APP_NOT_OWNED(Int = 4008)
— application not purchased.ERROR_INTERNAL_ERROR(Int = 4009)
— internal error.ERROR_ABORTED(Int = 4010)
— user refused to install the update.ERROR_APK_NOT_FOUND(Int = 4011)
— APK for installation not found.ERROR_EXTERNAL_SOURCE_DENIED(Int = 4012)
— update prohibited. For example, the first method responses that an update is not available, but the user calls the second method.ERROR_ACTIVITY_SEND_INTENT(Int = 9901)
— error while sending intent for opening an activity.ERROR_ACTIVITY_UNKNOWN(Int = 9902)
— unknown error on activity opening.