SDK Universal Push Notifications for Kotlin (version 2.0.0)
RuStore General Push Notifications SDK is a set of packages that allows you to work with multiple providers at the same time. You can send and receive messages via:
- RuStore
- Firebase Cloud Messaging(FCM)
- Huawei Mobile Services (HMS)
Use SDK together with pre-configured HMS and FCM.
Example implementation
See example appfor proper integration.
Settings for RuStore provider
Prerequisites
For push notifications to be sent via the RuStore provider, the following prerequisites must be met:
- The current version of RuStore is installed on the user's device.
- RuStore app supports push notifications.
- The RuStore app is allowed to run in the background.
- User is authorized in RuStore.
- The signature fingerprint of the app must match the fingerprint added to the RuStore Console.
Integrate into your project
Connect repository in settings.gradle
.
dependencyResolutionManagement {
repositories {
google()
mavenCentral()
// required for HMS
maven {url = uri("https://developer.huawei.com/repo/")}
// required for RuStore to work
maven {
url = uri("https://artifactory-external.vkpartner.ru/artifactory/maven")
}
}
}
Dependency injection
Add the following code to build.gradle
at the app
level to include dependencies.
dependencies {
implementation 'ru.rustore.sdk:universalpush:2.0.0'
implementation 'ru.rustore.sdk:universalrustore:2.0.0'
implementation 'ru.rustore.sdk:universalhms:2.0.0'
implementation 'ru.rustore.sdk:universalfcm:2.0.0'
}
Этого достаточно только для работы пакета ru.rustore.sdk:universalrustore
.
Для работы FCM и HMS выполните дополнительные шаги:
-
Add the following code to
build.gradle
at theapp
level.build.gradleplugins {
// ...
// нужно для FCM
id 'com.google.gms.google-services'
// нужно для HMS
id 'com.huawei.agconnect'
} -
Add the following code to
build.gradle
at the root level.build.gradledependencies {
// нужно для FCM
classpath 'com.google.gms:google-services:4.3.15'
// нужно для HMS
classpath 'com.huawei.agconnect:agcp:1.6.0.300'
classpath 'com.android.tools.build:gradle:7.4.0' -
Add the following code to
settings.gradle
at the root level.settings.gradlepluginManagement {
repositories {
google()
mavenCentral()
gradlePluginPortal()
// нужно для работы HMS
maven {url = uri("https://developer.huawei.com/repo/")}
}
}
Settings for FCM
Customising the app
To push notifications through via FCM provider, configure your project in Firebase Console.
-
Create a new project in console.firebase.google.com.
-
Select the project for which you want to enable push notifications.
-
Click the cog icon in the left menu beside the project name.
-
Go to Project Settings — Your apps and download
google-services.json
. -
Save
google-services.json
toapp/google-services.json
.
Integrate into your project
Connect the repository in settings.gradle
at the root level.
dependencyResolutionManagement {
repositories {
google()
mavenCentral()
// required for RuStore to work
maven {
url = uri("https://artifactory-external.vkpartner.ru/artifactory/maven")
}
}
}
Dependency injection
Add dependencies for push notifications to work only via FCM and RuStore to publish the app to Google Play.
You only have to use the universalfcm
, universalrustore
and universalpush
packages.
-
Add the following code to
build.gradle
at theapp
level.build.gradledependencies {
implementation 'ru.rustore.sdk:universalpush:2.0.0'
implementation 'ru.rustore.sdk:universalrustore:2.0.0'
implementation 'ru.rustore.sdk:universalfcm:2.0.0'
} -
Add the following code to
build.gradle
at theapp
level.build.gradleplugins {
// ...
// required for FCM
id 'com.google.gms.google-services'
} -
Add the following code to
build.gradle
at the root level.build.gradlebuildscript {
dependencies {
// required for FCM
classpath 'com.google.gms:google-services:4.3.15'
}
}
Settings for HMS
Customising the app
For push notifications to be transmitted via HMS provider, configure the project in developer.huawei.com.
-
Create a new project in developer.huawei.com.
-
Select the project for which you want to enable push notifications.
-
Go to Settings - General.
-
Go to Application Data and download
agconnect-services.json
. -
Save
agconnect-services.json
toapp/agconnect-services.json
.
Integrate into your project
Connect the repository in settings.gradle
.
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
// required for HMS
maven {url = uri("https://developer.huawei.com/repo/")}
// required for RuStore to work
maven {
url = uri("https://artifactory-external.vkpartner.ru/artifactory/maven")
}
}
}
Dependency injection
Add dependencies for push notifications to work only via HMS and RuStore to publish the app to AppGallery.
You only need to use universalhms
, universalrustore
, and universalpush
packages.
-
Add the following code to
build.gradle
at theapp
level.build.gradledependencies {
implementation 'ru.rustore.sdk:universalpush:2.0.0'
implementation 'ru.rustore.sdk:universalrustore:2.0.0'
implementation 'ru.rustore.sdk:universalhms:2.0.0'
} -
Add the following code to
build.gradle
at theapp
level.build.gradleplugins {
// ...
// нужно для HMS
id 'com.huawei.agconnect'
} -
Add the following code to
build.gradle
at the root level.build.gradlebuildscript {
dependencies {
// нужно для HMS
classpath 'com.huawei.agconnect:agcp:1.6.0.300'
classpath 'com.android.tools.build:gradle:7.4.0'
}
} -
Add the following code to
settings.gradle
at the root level.settings.gradlepluginManagement {
repositories {
google()
mavenCentral()
gradlePluginPortal()
// нужно для работы HMS
maven {url = uri("https://developer.huawei.com/repo/")}
}
}
SDK Initialization
For initialisation you will need project ID from the RuStore Console. To get the project ID on the app page navigate to Push notifications > Projects and copy the value in the Project ID field.
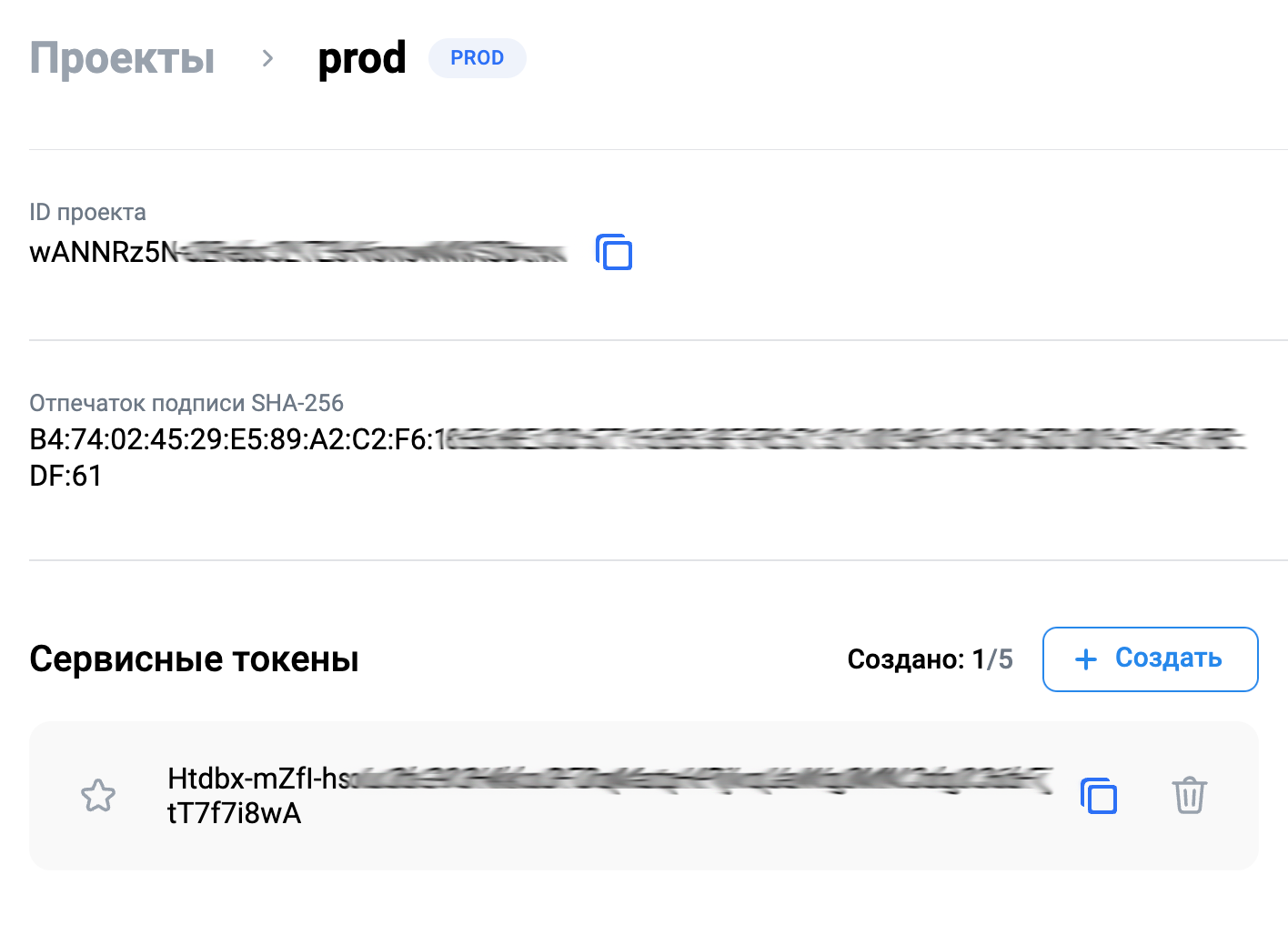
To initialise push notification providers, add the following code to App.kt
.
import android.app.Application
import ru.rustore.sdk.universalpush.RuStoreUniversalPushClient
import ru.rustore.sdk.universalpush.firebase.provides.FirebasePushProvider
import ru.rustore.sdk.universalpush.hms.providers.HmsPushProvider
import ru.rustore.sdk.universalpush.rustore.logger.DefaultLogger
import ru.rustore.sdk.universalpush.rustore.providers.RuStorePushProvider
class App: Application() {
private val tag = "UniversalPushExampleApp"
override fun onCreate() {
super.onCreate()
RuStoreUniversalPushClient.init(
context = this,
rustore = RuStorePushProvider(
application = this,
projectId = "m3Id6aPeXq36mp...",
logger = DefaultLogger(tag = tag),
),
firebase = FirebasePushProvider(
context = this,
),
hms = HmsPushProvider(
context = this,
appid = "108003365",
),
)
}
}
If you do not use all of the providers, initialise only the ones that you need. For example, the code below initialises only RuStore and HMS providers.
RuStoreUniversalPushClient.init(
context = this,
rustore = RuStorePushProvider(
application = this,
projectId = "m3Id6aPeXq36mp...",
logger = DefaultLogger(tag = tag),
),
hms = HmsPushProvider(
context = this,
appid = "108003365",
),
)
Performance test
To check push provider availability, call the checkAvailability (context)
method.
RuStoreUniversalPushClient.checkAvailability(this)
.addOnSuccessListener { result ->
Log.w(tag, "get availability success $result")
}
.addOnFailureListener { throwable ->
Log.e(tag, "get availability error: $throwable")
}
result
— key dictionary:
public const val UNIVERSAL_FCM_PROVIDER: String = "firebase"
public const val UNIVERSAL_HMS_PROVIDER: String = "hms"
public const val UNIVERSAL_RUSTORE_PROVIDER: String = "rustore"
You can check the specific provider as follows:
if (result[UNIVERSAL_HMS_PROVIDER] ?: false) {
// HMS provider available
}
Push token methods
Retrive user's push token
Чтобы получить список токенов по всем провайдерам, вызовите метод getTokens()
.
RuStoreUniversalPushClient.getTokens()
.addOnSuccessListener { result ->
Log.w(tag, "get tokens success $result")
}
.addOnFailureListener { throwable ->
Log.e(tag, "get tokens error: $throwable")
}
result
— key dictionary:
public const val UNIVERSAL_FCM_PROVIDER: String = "firebase"
public const val UNIVERSAL_HMS_PROVIDER: String = "hms"
public const val UNIVERSAL_RUSTORE_PROVIDER: String = "rustore"
You can get a specific token as follows:
result[UNIVERSAL_FCM_PROVIDER].orEmpty()
Delete user's push token
To delete tokens, call the deleteTokens(token)
method and pass a dictionary with a list of tokens.
RuStoreUniversalPushClient.deleteTokens(
mapOf(
UNIVERSAL_RUSTORE_PROVIDER to "xxx",
UNIVERSAL_FCM_PROVIDER to "yyy",
UNIVERSAL_HMS_PROVIDER to "zzz"
)
)
xxx
, yyy
, zzz
— tokens of push notification providers.
Push topic methods
Subscribe to topic-based push notifications
To subscribe to a topic, call the subscribeToTopic ("some_topic")
method.
RuStoreUniversalPushClient.subscribeToTopic("some_topic")
Unsubscribe from topic-based push notifications
To unsubscribe from a topic, call the unsubscribeToTopic ("some_topic")
method.
RuStoreUniversalPushClient.unsubscribeFromTopic("some_topic")
Notification handling
To receive notifications, add a OnMessageReceiveListener
callback in the App
class after the RuStoreUniversalPushClient
has been initialised.
When notifications are sent via universal api
, they are de-duplicated on the client. The receive notification callback is called once.
RuStore SDK will display the notification if there is data in notification
object.
If you don’t want RuStoreSDK to display notification itself, use data
object, and leave notification
object empty.
OnMessageReceiveListener
is called anyway.
You can get payload
push notifications (Map<String, String>
) from the remoteMessage.data
field.
RuStoreUniversalPushClient.setOnMessageReceiveListener { remoteMessage ->
// process message
}
Handle onDeletedMessages events
To handle the onDeletedMessages
event, add a OnDeletedMessagesListener
callback in the App
-class after the RuStoreUniversalPushClient
has been initialized.
The onDeletedMessages
event calls a callback with the parameter providerType
— push notification provider that triggered the event .
RuStoreUniversalPushClient.setOnDeletedMessagesListener { providerType ->
// process event
}
Handle onNewToken events
To handle the onNewToken
event, add a OnNewTokenListener
callback in the App
-class after the RuStoreUniversalPushClient
has been initialized.
In case of the onNewToken
event, a callback is invoked as follows:
providerType
— push notification provider that triggered the event .token
— new push token.
RuStoreUniversalPushClient.setOnNewTokenListener { providerType, token ->
// process event
}
Handling provider errors
To handle errors, add a OnPushClientErrorListener
callback to the App
class after the RuStoreUniversalPushClient
has been initialised.
In the event of an error, a callback with parameters will be called:
providerType
— push notification provider that triggered the event .errors
— list of errors.
RuStoreUniversalPushClient.setOnPushClientErrorListener { providerType, error ->
// process error
}
If HMS or FCM already sends push notifications
If you want to connect the Universal Push Notifications SDK, but your application is already using FCM or HMS services, add additional code:
-
Code for FCM service
import ru.rustore.sdk.universalpush.rustore.messaging.toUniversalRemoteMessage
import ru.rustore.sdk.universalpush.UNIVERSAL_FCM_PROVIDER
class MyFirebaseMessagingService: FirebaseMessagingService() {
override fun onMessageReceived(message: RemoteMessage) {
super.onMessageReceived(message)
RuStoreUniversalPushManager.processMessage(message.toUniversalRemoteMessage())
}
override fun onNewToken(token: String) {
super.onNewToken(token)
RuStoreUniversalPushManager.processToken(UNIVERSAL_FCM_PROVIDER, token)
}
override fun onDeletedMessages() {
super.onDeletedMessages()
RuStoreUniversalPushManager.processDeletedMessages(UNIVERSAL_FCM_PROVIDER)
}
} -
Code for HMS
import ru.rustore.sdk.universalpush.rustore.messaging.toUniversalRemoteMessage
import ru.rustore.sdk.universalpush.UNIVERSAL_HMS_PROVIDER
class MyMessagePushService: HmsMessageService() {
override fun onMessageReceived(msg: RemoteMessage?) {
super.onMessageReceived(msg)
RuStoreUniversalPushManager.processMessage(UNIVERSAL_HMS_PROVIDER, msg.toUniversalRemoteMessage())
}
override fun onNewToken(token: String?) {
super.onNewToken(token)
RuStoreUniversalPushManager.processToken(token)
}
override fun onDeletedMessages() {
super.onDeletedMessages()
RuStoreUniversalPushManager.processDeletedMessages(UNIVERSAL_HMS_PROVIDER)
}
}