Push notifications SDK for Defold (version 1.1.0)
Prerequisites
Push notification terms and conditions:
- The current version of RuStore is installed on the user's device.
- RuStore app supports push notifications.
- The RuStore app is allowed to run in the background.
- User is authorized in RuStore.
- The signature fingerprint of the app must match the fingerprint added to the RuStore Console.
Implementation example
Checkout example app to learn how to implement push notification SDK.
Connecting to project
Package installation
The extension-rustore-push
extension can be installed via a dependency connection and locally. Local installation allows you to modify project source and edit application manifest to fine-tune the SDK.
** Installation via dependencies **
The Defold User Guide on the Library page describes the rules for the connection of external dependencies.
-
Open your project settings in
Project
(game.project → Project); -
Add a reference to the package with the extension https://gitflic.ru/project/rustore/defold-extension-rustore-push/file/downloadAll?branch=master in the
Dependencies
field.
A reference to a specific release of the extension is recommended.
** Local installation (code modifiable) **
-
Copy the plugin project and example application from the official RuStore repository to GitFlic;
-
Copy the
extension-rustore-push
folder to the root of your project; -
Open your project settings in
Library
(game.project → Library); -
In the
Include Dirs
field, specify the name of theextension-rustore-push
package folder, with multiple names separated by spaces.
Editing app manifest
The extension-rustore-push
plugin will make changes to your application manifest.
You can change this behaviour in a local copy of extension-rustore-push
in the manifest file extension-rustore-push / manifests / android / Android / AndroidManifest.xml
.
The plugin will add the RustoreMessagingService
to your application manifest.
<service
android:name="ru.rustore.defoldpush.RustoreMessagingService"
android:exported="true"
tools:ignore="ExportedService">
<intent-filter>
<action android:name="ru.rustore.sdk.pushclient.MESSAGING_EVENT" />
</intent-filter>
</service>
To specify push client initialisation parameters, the plugin will add the project_id
and params_class
parameters to your app manifest via the meta-data
tag. For more information on project_id
and params_class
, see Initialisation.
<meta-data
android:name="ru.rustore.sdk.pushclient.project_id"
android:value="{{android.rustore_project_id}}" />
<meta-data
android:name="ru.rustore.sdk.pushclient.params_class"
android:value="ru.rustore.defoldpush.RuStorePushClientParams" />
If you need to change the icon or colour of the standard notification, add:
<meta-data
android:name="ru.rustore.sdk.pushclient.default_notification_icon"
android:resource="@drawable/ic_baseline_android_24" />
<meta-data
android:name="ru.rustore.sdk.pushclient.default_notification_color"
android:resource="@color/your_favorite_color" />
If you need to override the notification channel, add:
<meta-data
android:name="ru.rustore.sdk.pushclient.default_notification_channel_id"
android:value="@string/pushes_notification_channel_id" />
You must create the channel yourself if you add a push notification channel.
Requesting permissions for displaying notifications in Android 13+
The Android 13 version has a new permission to display push notifications. This will affect all apps that run on Android 13 or higher and use RuStore Push SDK.
By default, RuStore Push SDK version 1.4.0 and above includes the POST_NOTIFICATIONS
permission defined in the manifest.
However, the application also needs to request this permission at runtime via the android.permission.POST_NOTIFICATIONS
constant.
The app will only be able to show push notifications if the user grants a permission.
Request permission to show push notifications:
// Declare the launcher at the top of your Activity/Fragment:
private final ActivityResultLauncher<String> requestPermissionLauncher =
registerForActivityResult(new ActivityResultContracts.RequestPermission(), isGranted -> {
if (isGranted) {
// RuStore Push SDK (and your app) can post notifications.
} else {
// TODO: Inform user that your app will not show notifications.
}
});
private void askNotificationPermission() {
// This is only necessary for API level>= 33 (TIRAMISU)
if (Build.VERSION.SDK_INT>= Build.VERSION_CODES.TIRAMISU) {
if (ContextCompat.checkSelfPermission(this, Manifest.permission.POST_NOTIFICATIONS) ==
PackageManager.PERMISSION_GRANTED) {
// RuStore Push SDK (and your app) can post notifications.
} else if (shouldShowRequestPermissionRationale(Manifest.permission.POST_NOTIFICATIONS)) {
// TODO: display an educational UI explaining to the user the features that will be enabled
// by them granting the POST_NOTIFICATION permission. This UI should provide the user
// "OK" and "No thanks" buttons. If the user selects "OK," directly request the permission.
// If the user selects "No thanks," allow the user to continue without notifications.
} else {
// Directly ask for the permission
requestPermissionLauncher.launch(Manifest.permission.POST_NOTIFICATIONS);
}
}
}
Initialization
For initialisation you will need project ID from the RuStore Console. To get the project ID on the app page navigate to Push notifications > Projects and copy the value in the Project ID field.
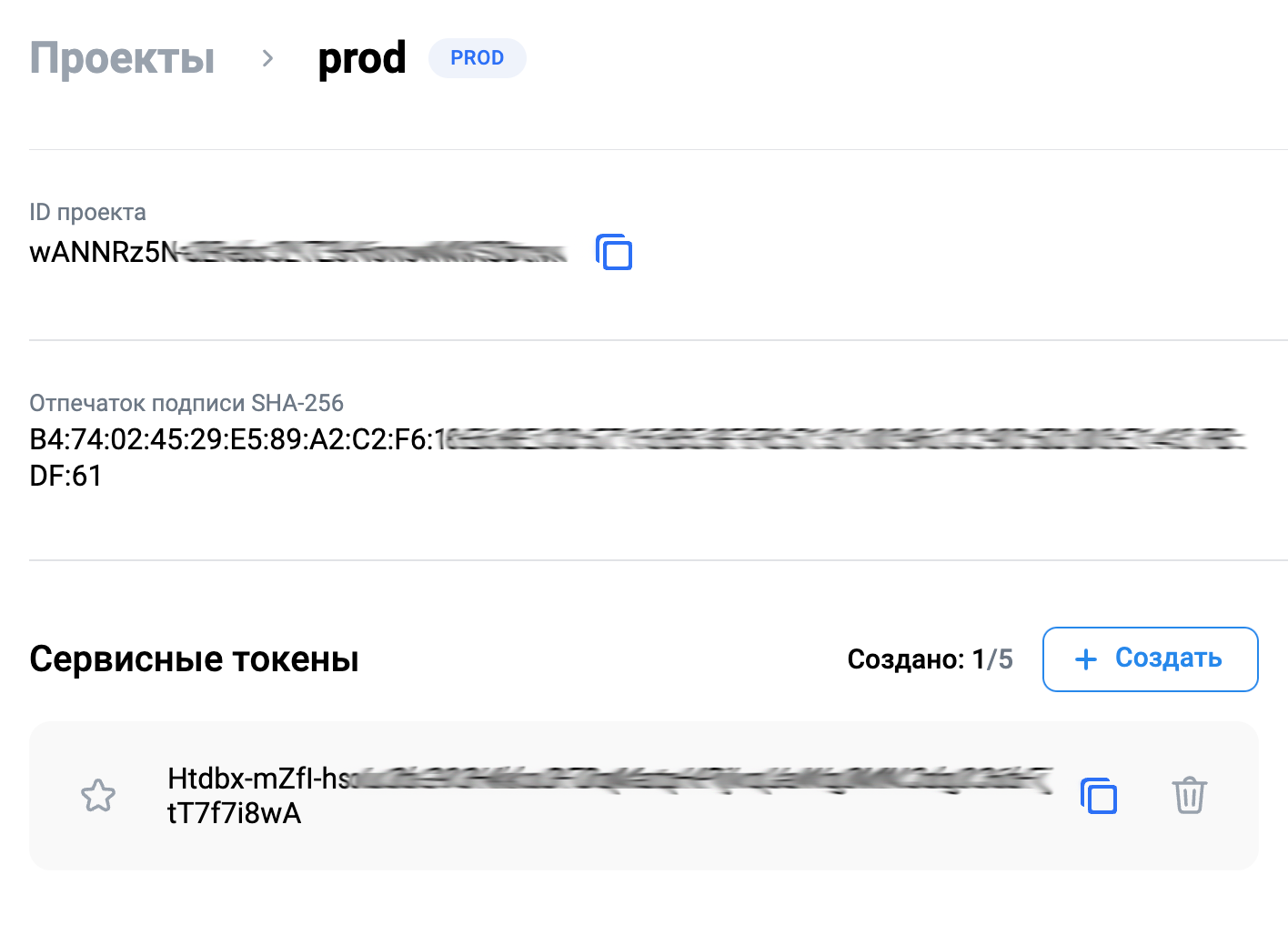
Plugin initialization
The plugin will add the following code to your app manifest:
You can change this behaviour in a local copy of extension-rustore-push
in the manifest file extension-rustore-push / manifests / android / Android / AndroidManifest.xml
.
<meta-data
android:name="ru.rustore.sdk.pushclient.project_id"
android:value="{{android.rustore_project_id}}" />
<meta-data
android:name="ru.rustore.sdk.pushclient.params_class"
android:value="ru.rustore.defoldpush.RuStorePushClientParams" />
-
projectId
— your project ID from RuStore Console. To get it, go to "Push Notifications" - "Projects" and copy the value in the "Project ID" field. -
params_class
(optional) — full class name of itsAbstractRuStorePushClientParams
implementation. It is required to specify additional parameters for push client initialisation.
An example of the successor to AbstractRuStorePushClientParams
is stored in extension-rustore-push / src / java / ru / rustore / defoldpush / RuStorePushClientParams.java
:
package ru.rustore.defoldpush;
import java.util.HashMap;
import java.util.Map;
import android.content.Context;
import com.vk.push.common.clientid.ClientIdCallback;
import ru.rustore.sdk.pushclient.common.logger.DefaultLogger;
import ru.rustore.sdk.pushclient.common.logger.Logger;
import ru.rustore.sdk.pushclient.provider.AbstractRuStorePushClientParams;
public class RuStorePushClientParams extends AbstractRuStorePushClientParams {
RuStorePushClientParams(Context context) {
super(context);
}
@Override
public Logger getLogger() {
return new DefaultLogger(Push.TAG);
}
@Override
public boolean getTestModeEnabled() {
return false;
}
@Override
public Map<String, Object> getInternalConfig() {
HashMap<String, Object> map = new HashMap<>(1);
map.put("type", "defold");
return map;
}
@Override
public ClientIdCallback getClientIdCallback() {
return Push.getInstance();
}
}
The implementation of the AbstractRuStorePushClientParams
class should have only one constructor with one Context argument.
To initialise the push notification service, add a value to game_project
of your project.
Open it with your favourite text editor, then add it to your [android]
section:
[android]
rustore_project_id = %your project id%
package = %your package%
your project id
— project ID from the RuStore Console. To get the project ID on the app page navigate to Push notifications > Projects and copy the value in the Project ID field.your package
— name of Android package from RuStore Console. To get it, go to "Push Notifications" - "Projects" and copy the value in the Android Package Name field.
Default header and body settings
You can set default header and body values if not specified in the push notification's data
field.
[android]
push_field_title = default push title
push_field_text = default push body
The push notification service starts automatically on Android devices when the set_on_token
method is called:
ruStorePush.set_on_token()
Event logging
Events of sdk push notifications are logged by default. An object implementing the Logger
interface is returned in getLogger
of RuStorePushClientParams
class, an implementation of the AbstractRuStorePushClientParams
abstract class. The SDK uses the default implementation with AndroidLog
if you don't pass Logger
:````
public interface Logger {
void verbose(String message, Throwable throwable);
void debug(String message, Throwable throwable);
void info(String message, Throwable throwable);
void warn(String message, Throwable throwable);
void error(String message, Throwable throwable);
Logger createLogger(String tag);
}
public class DefaultLogger implements Logger {
private final String tag;
public DefaultLogger(String tag) {
this.tag = tag;
}
@Override
public void debug( @NonNull String message, Throwable throwable) {
Log.d(tag, message, throwable);
}
@Override
public void error( @NonNull String message, Throwable throwable) {
Log.e(tag, message, throwable);
}
@Override
public void info( @NonNull String message, @Nullable Throwable throwable) {
Log.i(tag, message, throwable);
}
@Override
public void verbose( @NonNull String message, @Nullable Throwable throwable) {
Log.v(tag, message, throwable);
}
@Override
public void warn( @NonNull String message, @Nullable Throwable throwable) {
Log.w(tag, message, throwable);
}
@NonNull
@Override
public Logger createLogger( @NonNull String newTag) {
String combinedTag = (tag != null ) ? tag + ":" + newTag : newTag;
return new DefaultLogger(combinedTag);
}
}
package ru.rustore.defoldpush;
import android.content.Context;
import ru.rustore.sdk.pushclient.common.logger.DefaultLogger;
import ru.rustore.sdk.pushclient.common.logger.Logger;
import ru.rustore.sdk.pushclient.provider.AbstractRuStorePushClientParams;
public class RuStorePushClientParams extends AbstractRuStorePushClientParams {
RuStorePushClientParams(Context context) {
super(context);
}
@Override
public Logger getLogger() {
return new DefaultLogger(Push.TAG);
}
}
User segments
A segment is a group of users chosen based on specific criteria you set. For instance, it could include users who generate the most revenue or those using older versions of Android. Read more about the segments in MyTracker documentation.
To launch segments, use the set_client_id_callback
method with CLIENT_ID_VALUE
and CLIENT_ID_TYPE
parameters:
local CLIENT_ID_TYPE = ruStorePush.CLIENT_AID_NOT_AVAILABLE
local CLIENT_ID_VALUE = ""
ruStorePush.set_client_id_callback(function(self)
return CLIENT_ID_VALUE, CLIENT_ID_TYPE
end)
CLIENT_ID_TYPE
— ID type:CLIENT_AID_NOT_AVAILABLE
— advertising identifier is not used.CLIENT_GAID
— Google's advertising ID;CLIENT_OAID
— Huawei's advertising ID.
CLIENT_ID_VALUE
— ID value.
Push token methods
Getting user push token
To get push tokens, subscribe to the new_token
event using the set_on_token
method:
local function new_token(self, token, error)
if token then
print(token)
else
print(error.error)
end
end
local function push_android()
ruStorePush.set_on_token(new_token)
end
The method will create and return a new push token if the user does not have one.
Deleting user push token
To remove push tokens, use the delete_token
method:
ruStorePush.delete_token(function (self, error)
if error then
print("Error deleting token: %s", error.error)
else
print("push token deleted")
end
end)
Push topic methods
Push topic subscription
To subscribe to a topic, use the topic_subscribe
method:
local TOPIC_NAME = "defold"
ruStorePush.topic_subscribe("defold", function (self, error)
if error then
print("Error to subscribe: %s", error.error)
else
print("subscribe to: defold")
end
end)
TOPIC_NAME
— topic name.
Unsubscribe from push topic
Use the topic_unsubscribe
method to unsubscribe from a topic's message:
local TOPIC_NAME = "defold"
ruStorePush.topic_unsubscribe(TOPIC_NAME, function (self, error)
if error then
print("Error to unsubscribe: %s", error.error)
else
print("unsubscribe from: defold")
end
end)
TOPIC_NAME
— topic name.
Retrieving push notification contents
To get details about push notifications, add callback ruStorePush.set_on_message().
For push notification info, subscribe to the on_message
event using the set_on_message
method:
local function listener(self, payload, activated)
-- The payload arrives here.
pprint(payload)
end
local function push_android()
ruStorePush.set_on_message(listener)
end
payload
(lua table) —data
field of push notification;activated
(bool) - information about the user going to the application via push notification.
Do not send push messages with notification
and android.notification.title
: They will be processed by RuStore and don’t operate properly, you can’t use them to go to the app.
Send data push with the following fields``
title
— for push title.message
— for push body..