2.0.0
General
RuStore In-app updates SDK enable users to be kept up to date with the latest app version on their device. This allows them to stay informed about any performance enhancements or bug fixes that have been implemented.
User scenario example
Additionally, the SDK offers the ability to notify users of a new version and provide an option to install it. The installation process can occur in the background, while the user can track the progress of the update.
See the example app to learn how to integrate Updates SDK correctly.
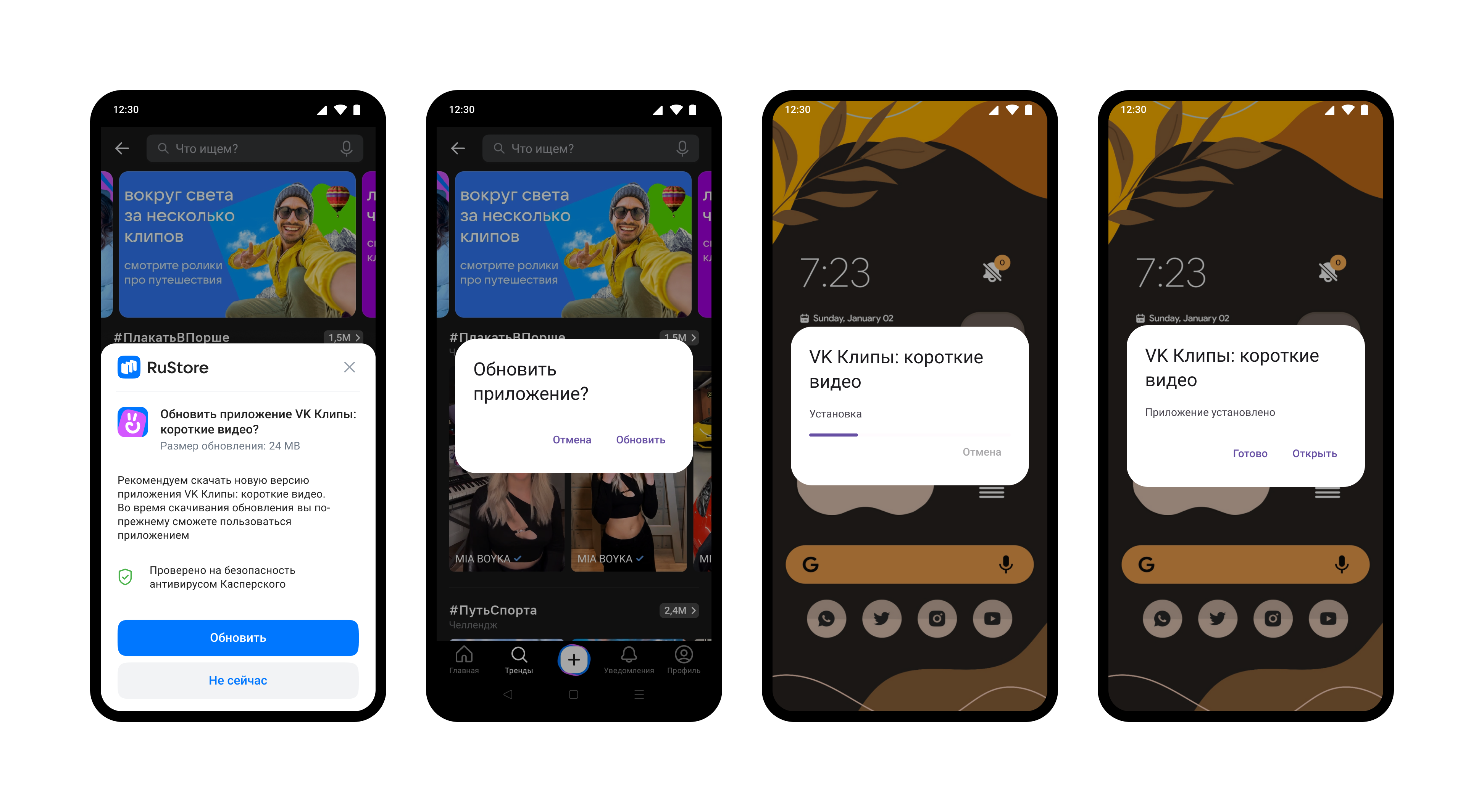
Prerequisites
For RuStore In-app updates SDK to operate correctly, the following conditions need to be met:
- Android 7.0 or later.
- RuStore app version on the device is up-to-date.
- The user is authorized in RuStore
- RuStore app is allowed to install applications
Connecting to project
To connect SDK to your project, you need to run the following code:
// HTTPS
npm install git+https://git@gitflic.ru/project/rustore/react-native-rustore-update-sdk.git
// SSH
npm install git+ssh://git@gitflic.ru/project/rustore/react-native-rustore-update-sdk.git
Создание менеджера обновлений
The SDK must be initialised for updates to work correctly.
RustoreUpdateClient.init();
Проверка наличия обновлений
Before requesting an update, check if it is available for your application To check for updates, call the getAppUpdateInfo()
method. When this method is called, the following conditions will be verified:
- The current version of RuStore is installed on the user's device.
- The user and the app should not be blocked in RuStore.
- RuStore app is allowed to install applications.
- The user is authorized in RuStore.
Upon calling this method, an appUpdateInfo object will be returned which contains information regarding any required updates.
try {
const appUpdateInfo = await RustoreUpdateClient.getAppUpdateInfo();
console.log(appUpdateInfo);
} catch (err) {
console.log(err);
}
AppUpdateInfo
object contains a set of parameters needed to determine if an update is available:
-
:updateAvailability
— update availability:UNKNOWN (int == 0)
— by default.;UPDATE_NOT_AVAILABLE (int == 1)
— no update required.;UPDATE_AVAILABLE (int == 2)
— update needs to be downloaded or it has already been downloaded to the user's device.;DEVELOPER_TRIGGERED_UPDATE_IN_PROGRESS (int == 3)
— update is already being downloaded or installation is already running..
-
:installStatus
— update installation status, if the user has already started the update installation at the time:UNKNOWN (int == 0)
— by default.;DOWNLOADED (int == 1)
— successfully downloaded.;DOWNLOADING (int == 2)
— currently being downloaded.;FAILED (int == 3)
— error.;PENDING (int == 5)
— awaiting update..
The update download is only available if the updateAvailability
field has the UPDATE_AVAILABLE
value.
Скачивание и установка обновлений
Использование слушателя (listener)
Once AppUpdateInfo
is received, you can check whether a push update is available.