1.0.1
General
RuStore In-app updates SDK enable users to be kept up to date with the latest app version on their device. This allows them to stay informed about any performance enhancements or bug fixes that have been implemented.
User scenario example
Additionally, the SDK offers the ability to notify users of a new version and provide an option to install it. The installation process can occur in the background, while the user can track the progress of the update.
See the example app to learn how to integrate Updates SDK correctly.
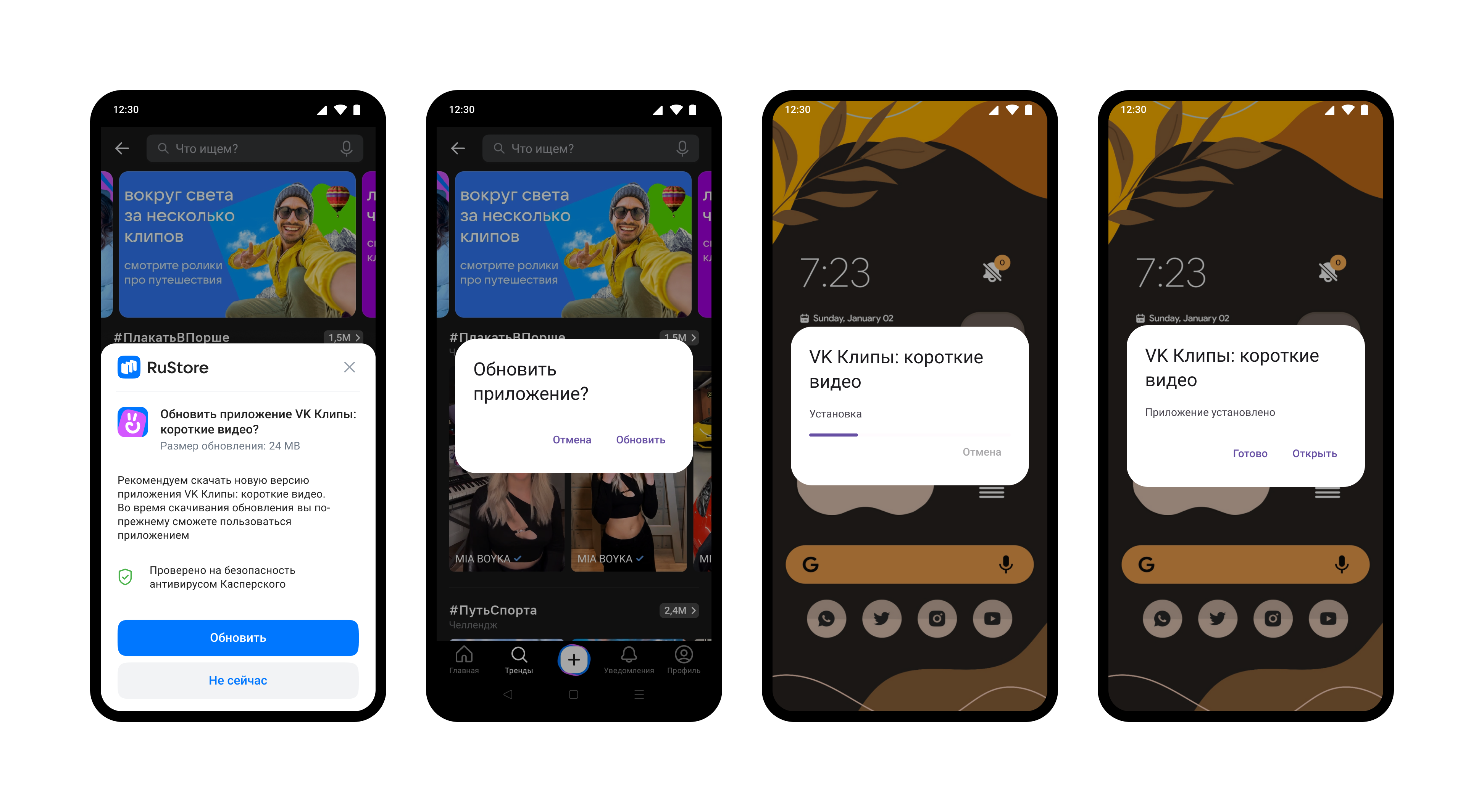
Prerequisites
For RuStore In-app updates SDK to operate correctly, the following conditions need to be met:
- Android 7.0 or later..
- RuStore app version on the device is up-to-date..
- The user is authorized in RuStore.
- RuStore app is allowed to install applications.
Connecting to project
To get started, follow these steps:
- Copy the plugin projects from the official RuStore repository to GitFlic.
- In your IDE, open the Android project from the
extension_libraries
folder. - Build your project with the command
gradle assemble
. If teh build is successful, the following files will be created inappupdate_example/extension_rustore_appupdate/lib/android
andappupdate_example/extension_rustore_core/lib/android
:RuStoreDefoldAppUpdate.jar
RuStoreDefoldCore.jar
- Copy the folders
appupdate_example/extension_rustore_appupdate
andappupdate_example/extension_rustore_core
to the root of your project.
Создание менеджера обновлений
Create update manager before calling library methods.
function init(self)
rustoreappupdate.init()
end
Проверка наличия обновлений
Before requesting an update, check if it is available for your application To check for updates, call the get_appupdateinfo
method. When this method is called, the following conditions will be verified:
- The current version of RuStore is installed on the user's device.
- The user and the app should not be blocked in RuStore.
- RuStore app is allowed to install applications.
- The user is authorized in RuStore.
You will need to subscribe to the events once before you can use this method:
rustore_get_app_update_info_success
;rustore_get_app_update_info_failure
.
function init(self)
rustorecore.connect("rustore_get_app_update_info_success", _on_get_app_update_info_success)
rustorecore.connect("rustore_get_app_update_info_failure", _on_get_app_update_info_failure)
-- Инициализация rustoreappupdate
end
function _on_get_app_update_info_success(self, channel, value)
local data = json.decode(value)
end
function _on_get_app_update_info_failure(self, channel, value)
local data = json.decode(value)
end
rustoreappupdate.get_appupdateinfo()
The rustore_get_app_update_info_success
callback returns строку JSON (объект AppUpdateInfo) with the update details. Request information in advance to run the update without delay at a convenient time for the user.
-
:updateAvailability
— update availability:UNKNOWN (int == 0)
— by default..UPDATE_NOT_AVAILABLE (int == 1)
— no update required..UPDATE_AVAILABLE (int == 2)
— update needs to be downloaded or it has already been downloaded to the user's device..DEVELOPER_TRIGGERED_UPDATE_IN_PROGRESS (int == 3)
— update is already being downloaded or installation is already running..
-
:installStatus
— update installation status, if the user has already started the update installation at the time:UNKNOWN (int == 0)
— by default..DOWNLOADED (int == 1)
— successfully downloaded..DOWNLOADING (int == 2)
— currently being downloaded..FAILED (int == 3)
— error..PENDING (int == 5)
— awaiting update..
-
availableVersionCode
— update version code.
rustore_get_app_update_info_failure
callback returns строку JSON with the error information. Error structure is described in Error Handling.
The update download is only available if the updateAvailability
field has the UPDATE_AVAILABLE
value.
Скачивание и установка обновлений
Использование слушателя
After the update's availability is confirmed, you can receive the update's download status. This involves subscribing to the on_state_updated
event and calling the register_listener
method to start the download status listener.
Проверка статуса скачивания обновления
You only need to subscribe to the events once rustore_on_state_updated
. Process listening is activated using the register_listener
method.
function init(self)
-- Initialization of rustoreappupdate
rustorecore.connect("rustore_on_state_updated", _on_state_updated)
rustoreappupdate.register_listener()
end
function _on_state_updated(self, channel, value)
local data = json.decode(value)
end
Therustore_on_state_updated
callback returns the json string with the update prompt. The object contains:
-
:installStatus
— update installation status, if the user has already started the update installation at the time:UNKNOWN (int == 0)
— by default..DOWNLOADED (int == 1)
— successfully downloaded..DOWNLOADING (int == 2)
— currently being downloaded..FAILED (int == 3)
— error..PENDING (int == 5)
— awaiting update..
-
.bytesDownloaded
— number of bytes downloaded. -
.totalBytesToDownload
— total number of bytes that need to be downloaded. -
packageName
— update package name.. -
installErrorCode
— error code during download. Error structure is described in Error Handling.
Удаление слушателя
If you no longer need a listener, use the unregister_listener
method.
rustoreappupdate.unregister_listener()
Запуск скачивания обновления
Отложенное обновление
Running an upgrade script
Update with RuStore UI
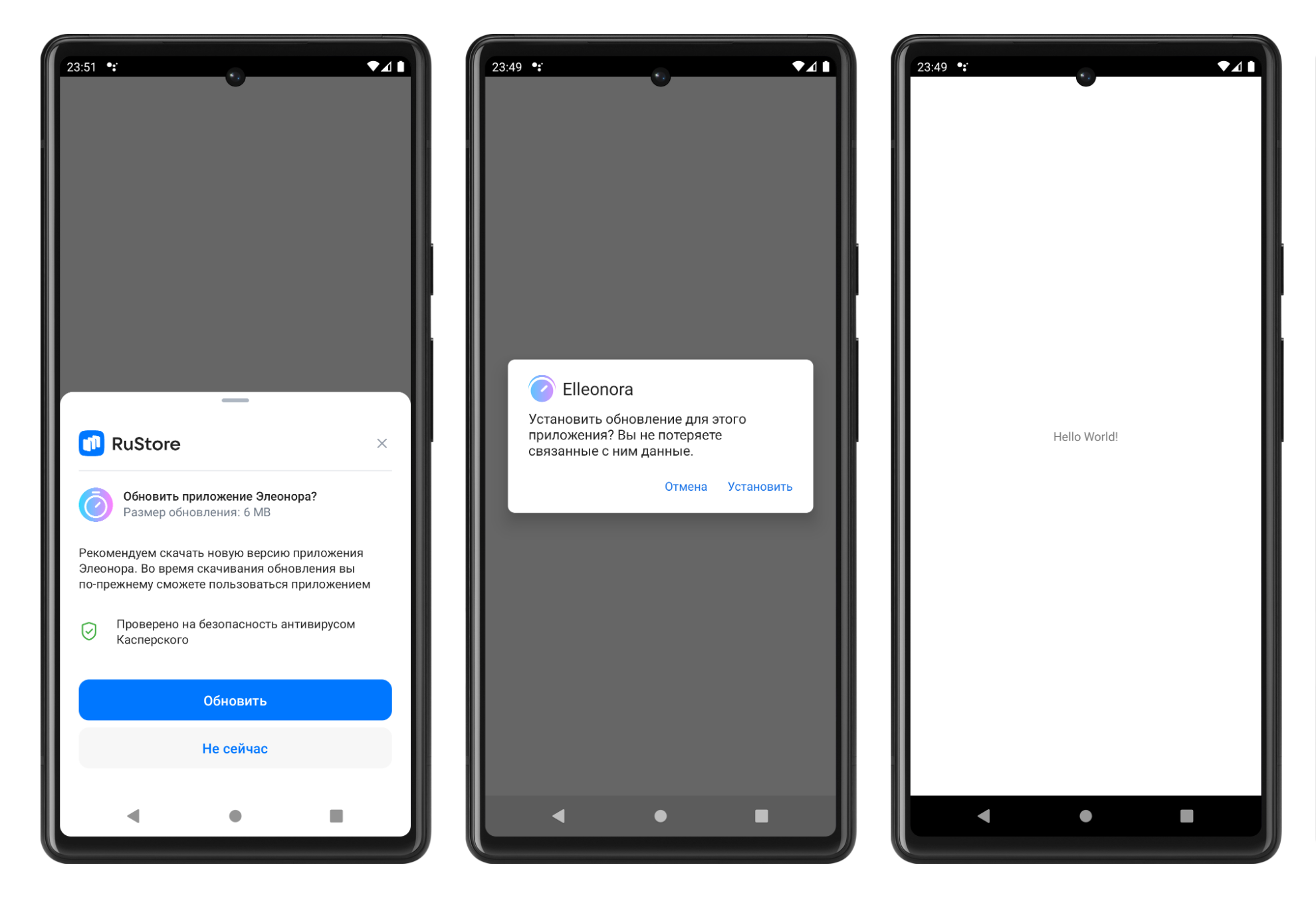
- To confirm the update, the user is presented with a RuStore UI dialogue.
- When you click the Update button, a dialogue box will appear to confirm that the update has been installed.
- If the update is successfully installed, the application will be closed.
Running an upgrade script
You will need to subscribe to the events once to start using this method:
rustore_start_update_flow_success
.rustore_start_update_flow_failure
.
To start the download, use start_update_flow_delayed
method.
It's important to note that the AppUpdateInfo
object becomes obsolete after a single use. Therefore, to call the startUpdateFlow()
method again, you should obtain a fresh AppUpdateInfo
instance by making another request to the getAppUpdateInfo()
method.
See Checking for updates. :::
function init(self)
rustorecore.connect("rustore_start_update_flow_success", _on_start_update_flow_success)
rustorecore.connect("rustore_start_update_flow_failure", _on_start_update_flow_failure)
-- Initialization of rustoreappupdate
end
function _on_start_update_flow_success(self, channel, value)
local data = json.decode(value)
end
function _on_start_update_flow_failure(self, channel, value)
local data = json.decode(value)
end
rustoreappupdate.start_update_flow_delayed()
The rustore_start_update_flow_success
callback returns a JSON string with information about the result of the update in the flowResult (number)
field:
RESULT_OK (int = -1)
— user has confirmed the download.RESULT_CANCELED (int = 0)
— user refused to install the update.
Therustore_start_update_flow_failure
callback returns the json string with the error details. All possible errors are described in Error Handling.
Once the DOWNLOADED (int == 1)
status is received in the installStatus
field of InstallState
, the install update method can be called.
Принудительное обновление
Running an upgrade script
Update with RuStore UI
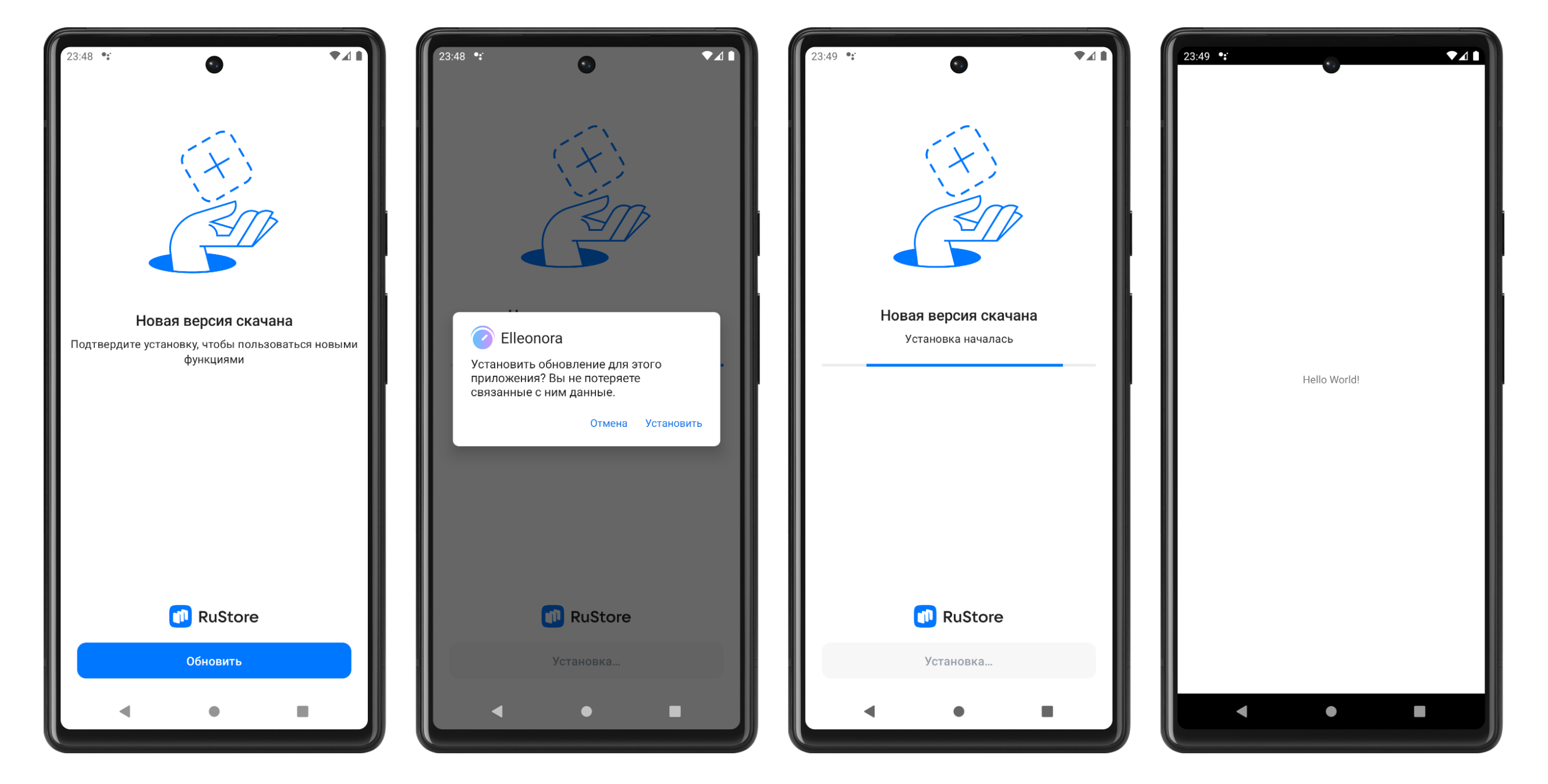
- To confirm the update, the user is presented with a RuStore UI dialogue. Until the update is installed, use of the application will be blocked.
- When you click the Update button, a dialogue box will appear to confirm that the update has been installed.
- If you then click on the 'Install' button, a full-screen dialogue box will appear asking you to install the new app version.
- Once the installation is complete, the application will restart.
If the Rustore version is 1.37 or higher, the application will restart. If the version of Rustore is lower, the application will close to install the update. It will not reopen when the update is complete.
Running an upgrade script
After receiving get_appupdateinfo
you can check the availability of the immediate update using the check_is_immediate_update_allowed
method.
local isAvailable = rustoreappupdate.check_is_immediate_update_allowed()
true
— forced update available;false
— forced update unavailable.
The result of check_is_immediate_update_allowed
is recommended when deciding whether to run a forced update, but this result does not affect the ability to run the script. Your internal app logic determines the necessity to run the update script.
You will need to subscribe to the events once to start using this method:
rustore_start_update_flow_success
.rustore_start_update_flow_failure
.
To start the download, use start_update_flow_immediate
method.
It's important to note that the AppUpdateInfo
object becomes obsolete after a single use. Therefore, to call the start_update_flow_immediate
method again, you should obtain a fresh AppUpdateInfo
instance by making another request to the get_appupdateinfo
method.
See Checking for updates. :::
function init(self)
rustorecore.connect("rustore_start_update_flow_success", _on_start_update_flow_success)
rustorecore.connect("rustore_start_update_flow_failure", _on_start_update_flow_failure)
-- Initialization of rustoreappupdate
end
function _on_start_update_flow_success(self, channel, value)
local data = json.decode(value)
end
function _on_start_update_flow_failure(self, channel, value)
local data = json.decode(value)
end
rustoreappupdate.start_update_flow_immediate()
The rustore_start_update_flow_success
callback returns a JSON string with information about the result of the update in the flowResult (number)
field:
RESULT_OK (int = -1)
— update completed, the code may not be received as the app terminates at the time of update.RESULT_CANCELED (int = 0)
— flow interrupted by user or an error occurred. It is expected, that the app should be stopped on receiving this code.ACTIVITY_NOT_FOUND (int = 2)
— RuStore is not installed, or an installed version does not support forced updating (RuStore versionCode
<191
).
Therustore_start_update_flow_failure
callback returns the json string with the error details. All possible errors are described in Error Handling.
No further action is required if the update is successful.
Тихое обновление
Silent Update Scenario Description
Update without RuStore UI
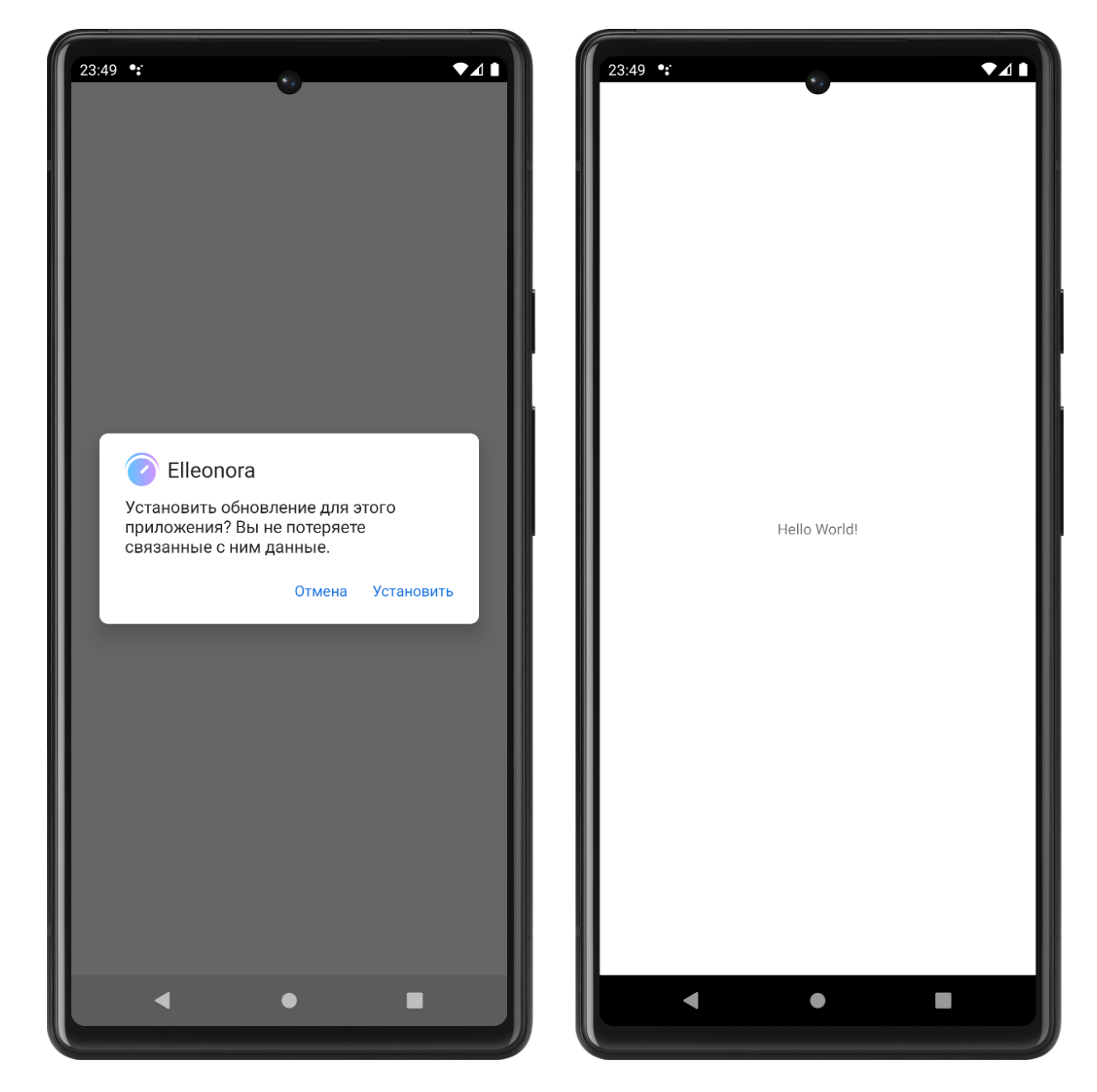
- The user will be presented with a dialogue box to confirm the installation of the update (the update will be downloaded in the background).
- If the update is successfully installed, the application will be closed.
Running an upgrade script
You will need to subscribe to the events once to start using this method:
rustore_start_update_flow_success
.rustore_start_update_flow_failure
.
To start the download, use start_update_flow_silent
method.
It's important to note that the AppUpdateInfo
object becomes obsolete after a single use. Therefore, to call the start_update_flow_immediate
method again, you should obtain a fresh AppUpdateInfo
instance by making another request to the get_appupdateinfo
method.
See Checking for updates. :::
function init(self)
rustorecore.connect("rustore_start_update_flow_success", _on_start_update_flow_success)
rustorecore.connect("rustore_start_update_flow_failure", _on_start_update_flow_failure)
-- Initialization of rustoreappupdate
end
function _on_start_update_flow_success(self, channel, value)
local data = json.decode(value)
end
function _on_start_update_flow_failure(self, channel, value)
local data = json.decode(value)
end
rustoreappupdate.start_update_flow_silent()
The rustore_start_update_flow_success
callback returns a JSON string with information about the result of the update in the flowResult (number)
field:
RESULT_OK (int = -1)
— task to download the update has been registered.
Therustore_start_update_flow_failure
callback returns the json string with the error details. All possible errors are described in Error Handling.
Call the install update method after the status DOWNLOADED (int == 1)
is received in the installStatus
field of the InstallState
object.
Silent update requires a separate interface to operate.
Установка обновления
It is advisable to notify the user that the update is prepared for installation at this point.
Once you have finished downloading the update file, you can run the update installation. To start the installation, use the complete_update
methods. You will need to subscribe to the events once before you can use this method:
rustore_complete_update_failure
.
function init(self)
rustorecore.connect("rustore_complete_update_failure", _on_complete_update_failure)
-- initialization of rustoreappupdate
end
function _on_complete_update_failure(self, channel, value)
local data = json.decode(value)
end
rustoreappupdate.complete_update()
The update is carried out through the native android tool. If the update is successfully installed, the application will be closed.
Therustore_complete_update_failure
callback returns the json string with the error details. All possible errors are described in Error Handling.
Errors processing
We do not recommend display an error to the user if you receive *_failure
in response. It can negatively affect the user experience.
function _on_failure(self, channel, value)
local data = json.decode(value)
local message = data.detailMessage
end
detailMessage
– error description.
Possible errors
RuStoreNotInstalledException
— RuStore not installed on user's device;RuStoreOutdatedException
— RuStore, installed on the user's device, does not support payment processing functions.;RuStoreUserUnauthorizedException
— user not authorized on RuStore;RuStoreException
— basic RuStore error, from which all other errors are inherited.;RuStoreInstallException(public val code: Int)
— download and installation error..ERROR_UNKNOWN(Int = 4001)
— unknown error.ERROR_DOWNLOAD(Int = 4002)
— error while downloading.ERROR_BLOCKED(Int = 4003)
— installation blocked by system.ERROR_INVALID_APK(Int = 4004)
— invalid update APK.ERROR_CONFLICT(Int = 4005)
— conflict with the current app version.ERROR_STORAGE(Int = 4006)
— insufficient device storage.ERROR_INCOMPATIBLE(Int = 4007)
— incompatible with device.ERROR_APP_NOT_OWNED(Int = 4008)
— application not purchased.ERROR_INTERNAL_ERROR(Int = 4009)
— internal error.ERROR_ABORTED(Int = 4010)
— user refused to install the update.ERROR_APK_NOT_FOUND(Int = 4011)
— APK for installation not found.ERROR_EXTERNAL_SOURCE_DENIED(Int = 4012)
— update prohibited. For example, the first method responses that an update is not available, but the user calls the second method.ERROR_ACTIVITY_SEND_INTENT(Int = 9901)
— error while sending intent for opening an activity.ERROR_ACTIVITY_UNKNOWN(Int = 9902)
— unknown error on activity opening.